Introduction
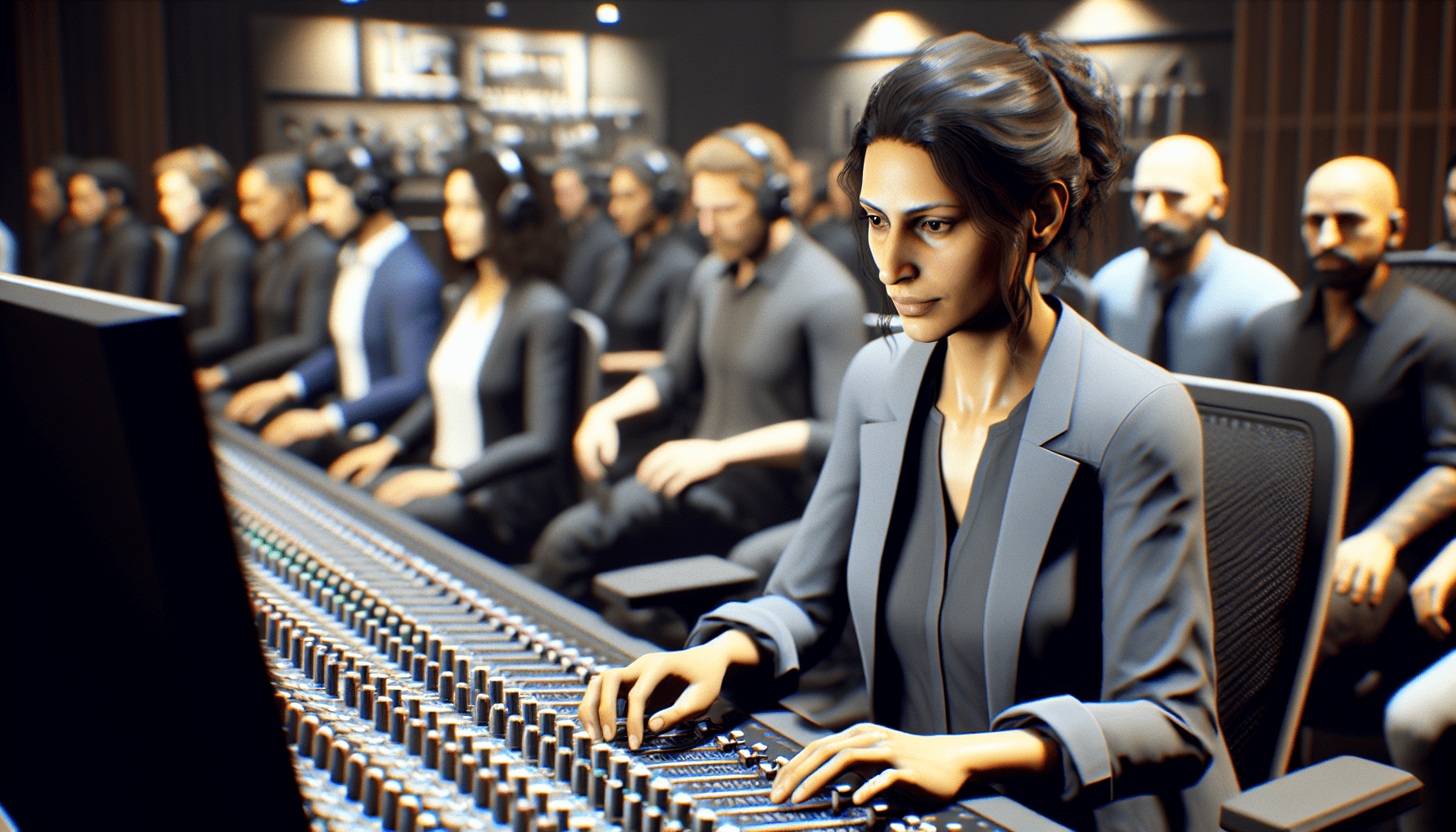
In the fast-paced world of video game development, sound design plays a crucial role in creating immersive experiences. Leveraging AI-driven sound design techniques in Unreal Engine can significantly enhance a game’s auditory landscape, making it more engaging and realistic.
This article explores the key aspects of AI sound design in Unreal Engine, addressing common challenges and providing practical solutions for game developers.
AI-Driven Sound Design in Unreal Engine
Key Points
- AI sound design enhances immersion and realism in games.
- Unreal Engine offers robust tools for integrating AI-driven audio.
- AI can dynamically adapt soundscapes based on gameplay.
- Efficient sound design can improve game performance.
- AI-driven techniques can reduce the need for extensive manual audio editing.
Overview of AI Sound Design
AI sound design in Unreal Engine involves using artificial intelligence to create, manipulate, and implement audio elements within a game. This approach allows for dynamic soundscapes that adapt to player actions and environmental changes. By leveraging AI, developers can automate complex audio tasks, such as generating ambient sounds or adjusting audio levels based on in-game events.
Unreal Engine provides a comprehensive suite of tools for integrating AI-driven audio. These tools include real-time audio mixing, advanced digital signal processing (DSP) effects, and adaptive music capabilities. Developers can use these features to create immersive soundscapes that enhance the player’s experience and interaction with the game world.
AI-driven sound design also offers significant performance benefits. By automating audio tasks, developers can reduce the need for extensive manual editing, freeing up resources for other aspects of game development. Additionally, AI can optimize audio performance by dynamically adjusting soundscapes based on gameplay, ensuring a seamless and engaging experience for players.
Benefits of AI Sound Design
One of the primary benefits of AI sound design is its ability to enhance immersion and realism in games. By dynamically adapting soundscapes based on player actions and environmental changes, AI-driven audio can create a more engaging and lifelike gaming experience. This level of immersion is crucial for modern games, where players expect high-quality audio that complements the visual and narrative elements of the game.
AI sound design also offers significant efficiency gains for developers. By automating complex audio tasks, AI can reduce the time and effort required for sound design, allowing developers to focus on other aspects of game development. This efficiency can be particularly beneficial for small to medium-sized studios, where resources may be limited.
Furthermore, AI-driven sound design can improve game performance by optimizing audio processing. By dynamically adjusting soundscapes based on gameplay, AI can ensure that audio resources are used efficiently, reducing the risk of performance issues and enhancing the overall player experience.
Challenges in AI Sound Design
Complexity of Dynamic Soundscapes
Creating dynamic soundscapes that adapt to player actions and environmental changes can be a complex task. Developers must consider various factors, such as the game’s narrative, visual elements, and player interactions, to ensure that the audio complements the overall gaming experience. This complexity can be challenging for developers, particularly those with limited experience in sound design.
Additionally, the integration of AI-driven audio tools can add another layer of complexity to the development process. Developers must be familiar with the capabilities and limitations of these tools to effectively implement AI-driven sound design techniques. This requires a deep understanding of both the technical and creative aspects of sound design, which can be a significant challenge for some developers.
Balancing Audio Performance and Quality
Another challenge in AI sound design is balancing audio performance and quality. While AI-driven techniques can optimize audio processing and improve performance, developers must ensure that these optimizations do not compromise the quality of the audio. This requires careful consideration of various factors, such as audio levels, soundscapes, and player interactions, to ensure that the audio enhances the overall gaming experience.
Developers must also consider the impact of AI-driven audio on the game’s performance. While AI can optimize audio processing, it can also introduce additional computational overhead, which can affect the game’s performance. Developers must carefully balance these factors to ensure that the audio enhances the gaming experience without negatively impacting performance.
Integration with Existing Game Systems
Integrating AI-driven sound design techniques with existing game systems can be a significant challenge for developers. This integration requires a deep understanding of both the game’s audio systems and the AI-driven tools used to create and manipulate audio elements. Developers must ensure that these systems work seamlessly together to create a cohesive and immersive gaming experience.
Additionally, developers must consider the impact of AI-driven audio on other aspects of the game, such as gameplay, narrative, and visual elements. This requires careful planning and coordination to ensure that the audio complements the overall gaming experience and enhances the player’s immersion and engagement.
Implementing AI Sound Design Solutions
Step 1: Understanding AI Audio Tools
To effectively implement AI sound design techniques, developers must first understand the capabilities and limitations of the AI audio tools available in Unreal Engine. This involves familiarizing themselves with the various features and functionalities of these tools, such as real-time audio mixing, DSP effects, and adaptive music capabilities.
Developers should also explore the integration options available for these tools, such as plugins and middleware, to ensure that they can seamlessly incorporate AI-driven audio into their game projects. By understanding these tools, developers can effectively leverage AI to create dynamic and immersive soundscapes that enhance the overall gaming experience.
Step 2: Designing Dynamic Soundscapes
Once developers have a solid understanding of the AI audio tools available, they can begin designing dynamic soundscapes that adapt to player actions and environmental changes. This involves creating audio elements that complement the game’s narrative, visual elements, and player interactions, ensuring that the audio enhances the overall gaming experience.
Developers should also consider the performance implications of their sound design, ensuring that the audio is optimized for efficient processing and does not negatively impact the game’s performance. By carefully designing dynamic soundscapes, developers can create immersive and engaging audio experiences that enhance the player’s immersion and engagement.
Step 3: Integrating AI-Driven Audio
The final step in implementing AI sound design techniques is integrating AI-driven audio into the game’s existing systems. This involves coordinating with other aspects of the game, such as gameplay, narrative, and visual elements, to ensure that the audio complements the overall gaming experience.
Developers should also consider the impact of AI-driven audio on the game’s performance, ensuring that the audio is optimized for efficient processing and does not introduce additional computational overhead. By effectively integrating AI-driven audio, developers can create a cohesive and immersive gaming experience that enhances the player’s immersion and engagement.
Code Example: Implementing AI Sound Design
In this section, we will provide a code example demonstrating how to implement AI sound design techniques in Unreal Engine. This example will use C++ to create a dynamic soundscape that adapts to player actions and environmental changes.
// Unreal Engine C++ code example for AI sound design
#include "AIController.h"
#include "Sound/SoundCue.h"
#include "Kismet/GameplayStatics.h"
#include "GameFramework/Actor.h"
class AMyAIController : public AAIController
{
public:
AMyAIController();
protected:
virtual void BeginPlay() override;
private:
UPROPERTY(EditAnywhere, Category = "Sound")
USoundCue* AmbientSoundCue;
UPROPERTY(EditAnywhere, Category = "Sound")
USoundCue* BattleSoundCue;
void PlayAmbientSound();
void PlayBattleSound();
};
AMyAIController::AMyAIController()
{
// Constructor logic
}
void AMyAIController::BeginPlay()
{
Super::BeginPlay();
PlayAmbientSound();
}
void AMyAIController::PlayAmbientSound()
{
if (AmbientSoundCue)
{
UGameplayStatics::PlaySoundAtLocation(this, AmbientSoundCue, GetActorLocation());
}
}
void AMyAIController::PlayBattleSound()
{
if (BattleSoundCue)
{
UGameplayStatics::PlaySoundAtLocation(this, BattleSoundCue, GetActorLocation());
}
}
Code language: C++ (cpp)
This code example demonstrates how to use Unreal Engine’s C++ API to implement AI sound design techniques. The AMyAIController
class extends the AAIController
class and includes methods for playing ambient and battle sounds. The BeginPlay
method is overridden to play the ambient sound when the game starts.
The PlayAmbientSound
and PlayBattleSound
methods use the UGameplayStatics::PlaySoundAtLocation
function to play the specified sound cues at the actor’s location. This allows the sound to dynamically adapt to the player’s actions and environmental changes, creating a more immersive and engaging audio experience.
FAQs
What is AI sound design in Unreal Engine?
AI sound design in Unreal Engine involves using artificial intelligence to create, manipulate, and implement audio elements within a game. This approach allows for dynamic soundscapes that adapt to player actions and environmental changes, enhancing the overall gaming experience.
How can AI improve sound design in games?
AI can improve sound design in games by automating complex audio tasks, optimizing audio performance, and creating dynamic soundscapes that adapt to gameplay. This enhances the player’s immersion and engagement, creating a more lifelike and engaging gaming experience.
What are the challenges of AI sound design?
The challenges of AI sound design include the complexity of creating dynamic soundscapes, balancing audio performance and quality, and integrating AI-driven audio with existing game systems. Developers must carefully consider these factors to ensure that the audio enhances the overall gaming experience.
How can developers implement AI sound design techniques?
Developers can implement AI sound design techniques by understanding the capabilities and limitations of AI audio tools, designing dynamic soundscapes that adapt to player actions and environmental changes, and integrating AI-driven audio into the game’s existing systems.
Future of AI Sound Design in Gaming
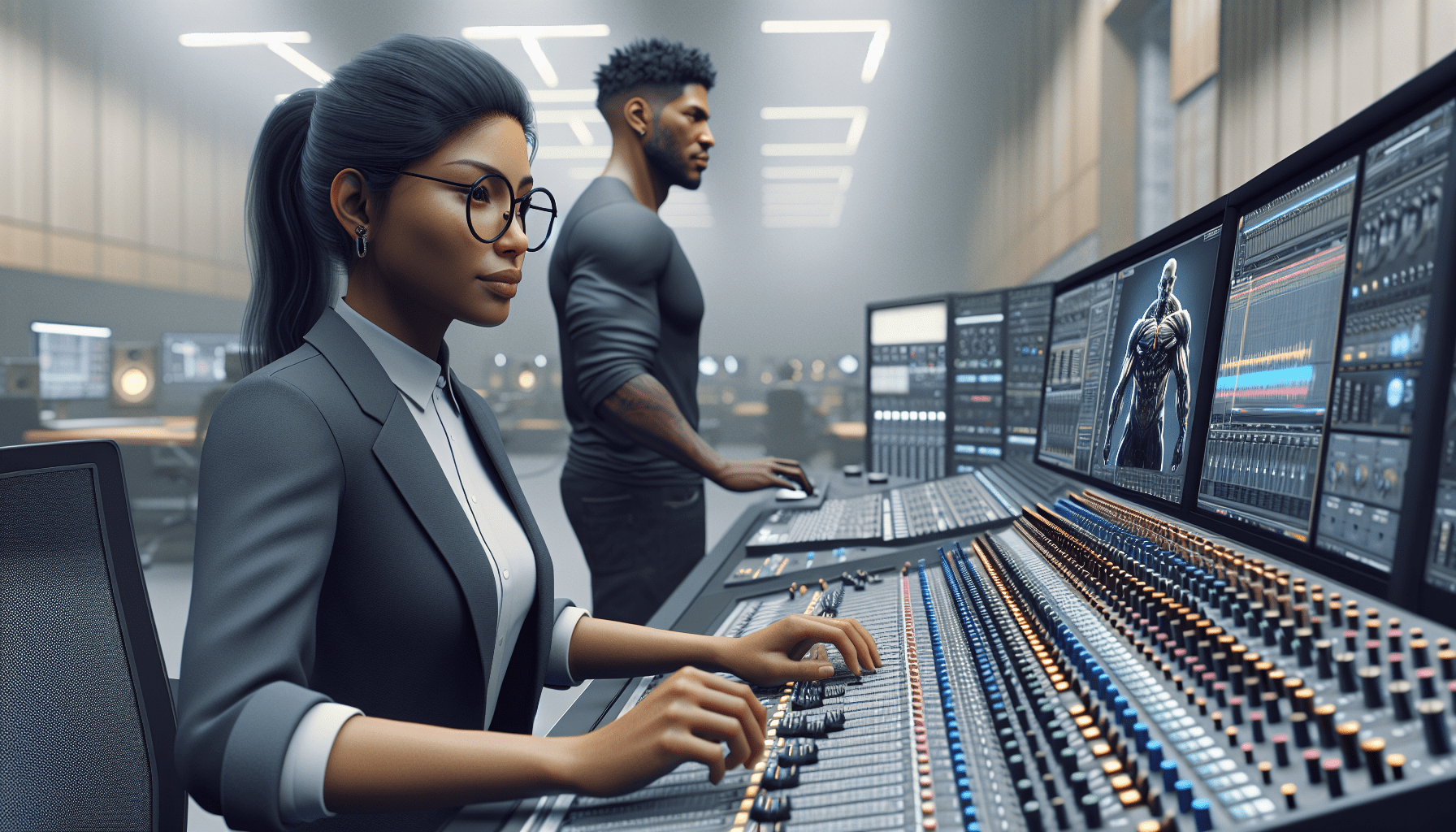
The future of AI sound design in gaming is promising, with several trends and advancements shaping the industry. Here are five predictions for the future of AI sound design in gaming:
- Increased use of AI-driven audio tools: As AI technology continues to advance, we can expect to see an increased use of AI-driven audio tools in game development, allowing for more dynamic and immersive soundscapes.
- Improved integration with game engines: AI sound design tools will become more seamlessly integrated with game engines, making it easier for developers to implement AI-driven audio techniques in their projects.
- Enhanced performance optimization: AI-driven audio tools will continue to improve performance optimization, allowing for more efficient audio processing and reducing the risk of performance issues.
- Greater focus on player immersion: AI sound design will increasingly focus on enhancing player immersion, creating more lifelike and engaging gaming experiences.
- Expansion of AI-driven audio capabilities: The capabilities of AI-driven audio tools will continue to expand, offering developers more options for creating dynamic and immersive soundscapes.
More Information
- Overlapping Audio called on in AI Controller BP(UE4 – v4.26.2) – Blueprint – Epic Developer Community Forums: A forum discussion on handling overlapping audio in Unreal Engine.
- Audio Programming in Unreal – Reddit: A Reddit thread discussing audio programming techniques in Unreal Engine.
- Enemy AI will not move towards noise event – AI – Epic Developer Community Forums: A forum discussion on AI behavior in response to noise events in Unreal Engine.
- How can I make my AI NPC’s have a footstep sound? – Game Development Stack Exchange: A Q&A on implementing footstep sounds for AI NPCs in games.
- Essential Sound Effects Tools for AAA Game Development in 2024 – Respeecher: An article discussing essential sound effects tools for AAA game development.
Disclaimer
This is an AI-generated article intended for educational purposes. It does not provide advice or recommendations for implementation. Readers are encouraged to conduct further research and explore the topics discussed in this article.
- Burnout in Remote Teams: How It’s Draining Your Profits - January 27, 2025
- Signs You’re Understaffed - January 20, 2025
- The Cost of Silence: Communicating Negative Feedback - January 13, 2025