Overview of Scalable UI Frameworks
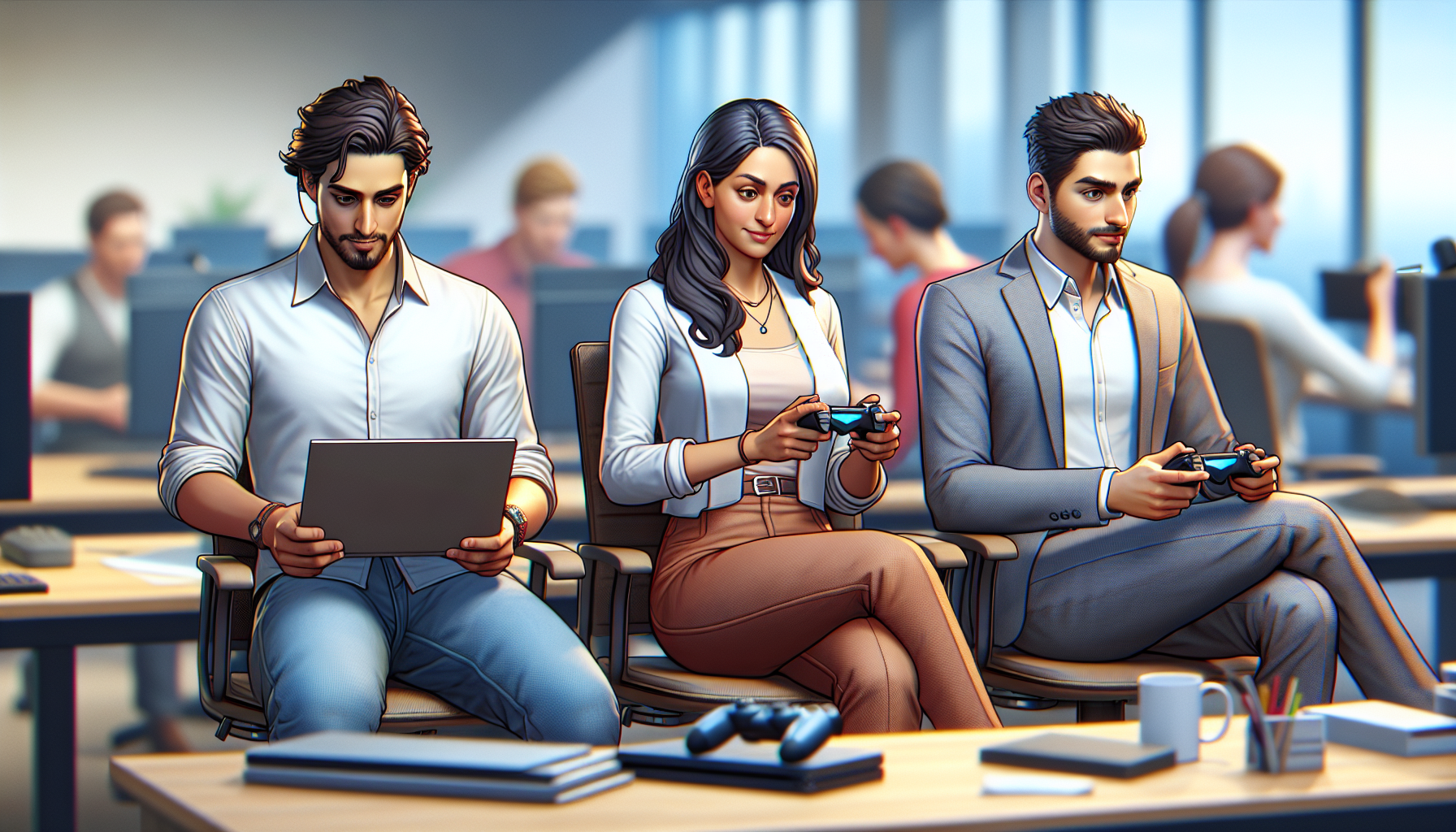
Key Points
- Low-resolution Unity UI scaling issues.
- Challenges with Canvas Scaler in high-resolution environments.
- Problems with rendering to a texture and mouse interaction.
- Frustrations with the tedious nature of UI development.
- Importance of UI for readability and functionality.
Definition and Importance
Scalable UI frameworks are essential in the development of multiplayer games. They ensure that the user interface (UI) adapts seamlessly to different screen sizes and resolutions. This adaptability is crucial for providing a consistent user experience across various devices, from mobile phones to high-resolution monitors. In multiplayer games, where players interact with each other in real-time, a scalable UI framework helps maintain the game’s usability and accessibility.
In the context of game development, a scalable UI framework involves designing UI elements that can dynamically adjust their size, position, and layout based on the screen’s resolution and aspect ratio. This approach minimizes the need for manual adjustments and ensures that the UI remains functional and visually appealing, regardless of the device being used.
Challenges in the Industry
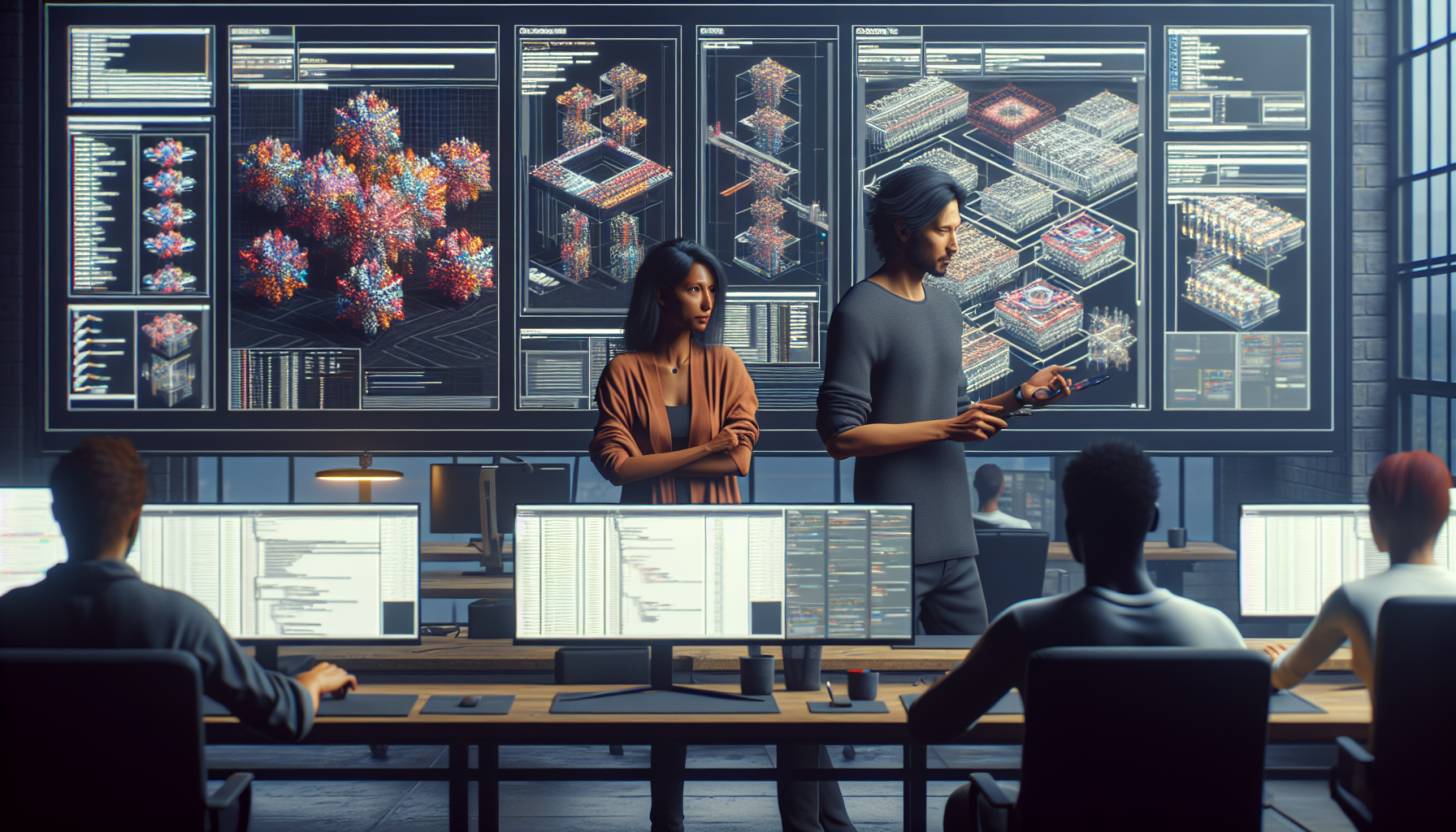
Complexity of Multiplayer UI Design
Designing a scalable UI for multiplayer games is inherently complex. The need to accommodate various screen sizes and resolutions adds layers of difficulty. Developers must ensure that the UI remains functional and visually appealing across all devices, from smartphones to high-resolution monitors. This complexity often leads to increased development time and costs, making it a significant challenge for small to medium-sized game development studios.
Moreover, multiplayer games require real-time interaction between players, which necessitates a responsive and intuitive UI. Players need to access various menus, chat windows, and in-game notifications quickly and efficiently. A poorly designed UI can lead to frustration and negatively impact the player’s experience, making it crucial for developers to prioritize UI design in multiplayer games.
Performance Optimization
Another major challenge in developing scalable UI frameworks for multiplayer games is performance optimization. UI elements can consume significant resources, especially in complex multiplayer games with numerous interactive components. Developers must optimize the UI to minimize its impact on the game’s performance, ensuring smooth gameplay even on lower-end devices.
Balancing the need for a visually appealing and functional UI with performance considerations is a delicate task. Developers must carefully manage resources and implement efficient rendering techniques to maintain the game’s performance. This challenge is particularly relevant for small to medium-sized game development studios, which may have limited resources and expertise in performance optimization.
Testing Across Devices
Testing the UI across different devices and resolutions is a time-consuming and resource-intensive process. Developers need access to a wide range of hardware to ensure that the UI performs well on all devices. Automated testing tools can help streamline this process, but they may not always capture the nuances of the user experience on different devices.
Additionally, manual testing is often required to identify and address specific issues that may arise on certain devices. Ensuring a consistent and high-quality user experience across all devices is a significant challenge in the development of scalable UI frameworks for multiplayer games.
Steps to Develop a Scalable UI Framework
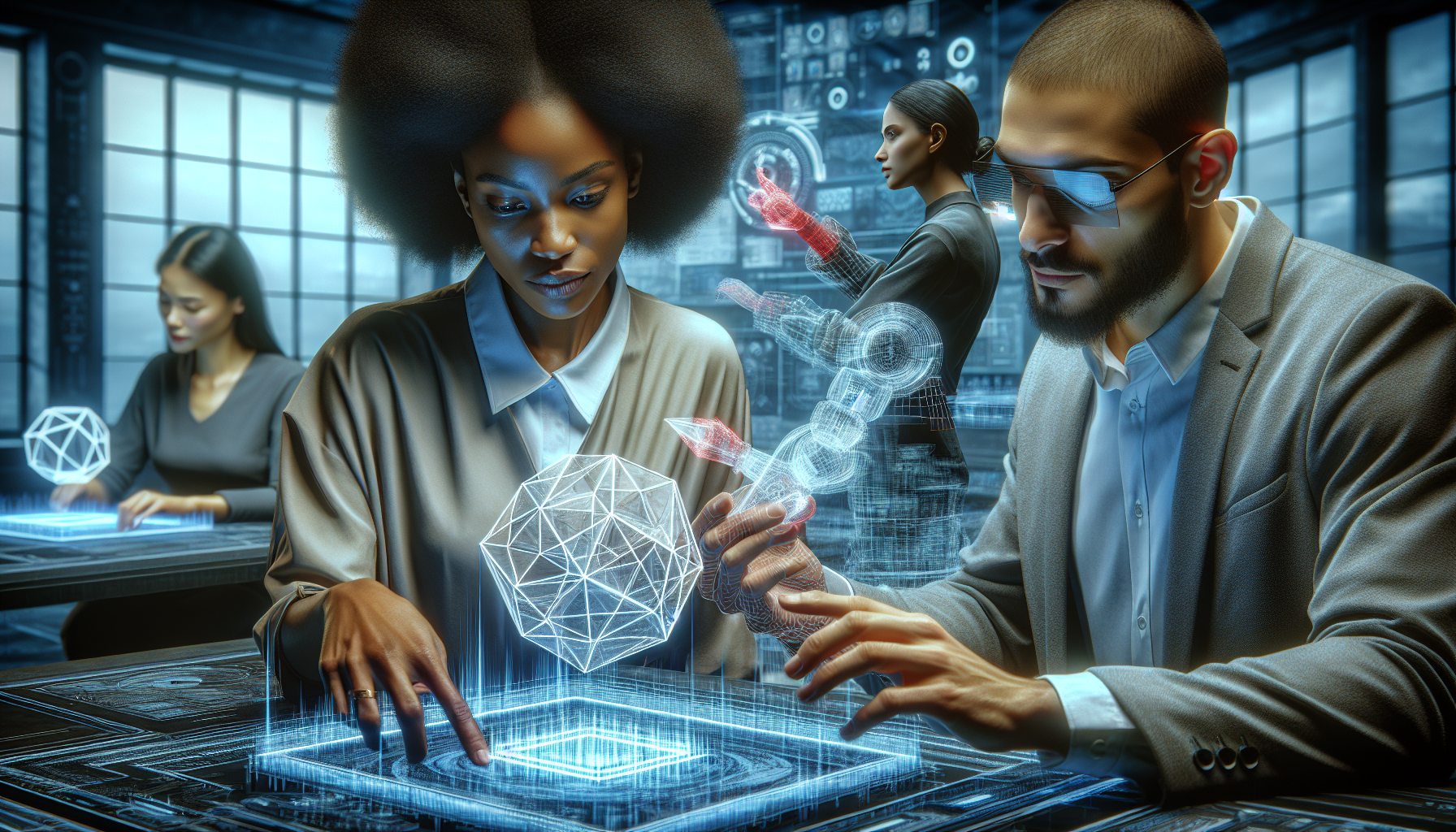
Step 1: Define UI Requirements
The first step in developing a scalable UI framework is to define the UI requirements. This involves understanding the target audience, the devices they use, and the specific needs of the multiplayer game. Developers should consider factors such as the game’s genre, the complexity of the UI elements, and the players’ interaction patterns.
By clearly defining the UI requirements, developers can create a roadmap for the UI design process. This roadmap should outline the key features and functionalities of the UI, as well as the design principles that will guide the development process.
Step 2: Design Responsive UI Elements
Once the UI requirements are defined, the next step is to design responsive UI elements. This involves creating flexible layouts that adapt to different screen sizes and resolutions. Developers should use techniques such as fluid grids, flexible images, and media queries to achieve a responsive design.
Additionally, using vector graphics for UI elements can help ensure that the UI remains sharp and clear on all devices. Vector graphics can scale without losing quality, making them ideal for scalable UI frameworks.
Step 3: Implement and Test the UI
After designing the responsive UI elements, the next step is to implement and test the UI. Developers should use a combination of automated and manual testing to ensure that the UI performs well on all devices. Automated testing tools can help identify and address common issues, while manual testing can provide valuable insights into the user experience on different devices.
Conducting usability tests with real players can provide valuable insights into how the UI performs and highlight any issues that need to be addressed. By incorporating user feedback, developers can create a more intuitive and user-friendly UI that enhances the overall gaming experience.
Code Example: Implementing a Scalable UI Framework in Unity
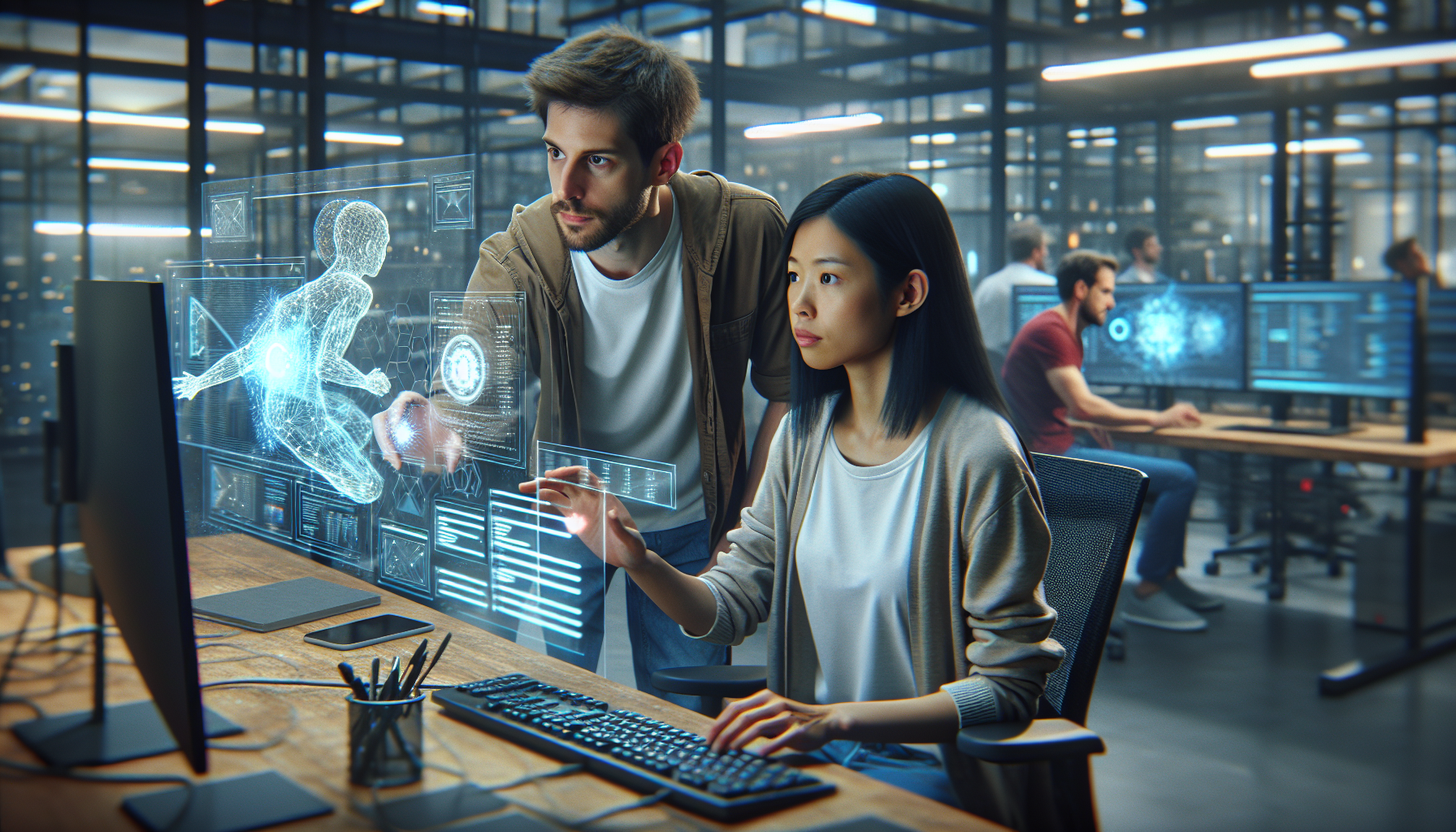
In this section, we will provide a code example of implementing a scalable UI framework in Unity. The example will demonstrate how to create responsive UI elements that adapt to different screen sizes and resolutions. We will use C# as the programming language and Unity’s built-in UI components.
using UnityEngine;
using UnityEngine.UI;
public class ScalableUI : MonoBehaviour
{
public CanvasScaler canvasScaler;
void Start()
{
ConfigureCanvasScaler();
}
/// <summary>
/// Configures the CanvasScaler to adapt to different screen sizes and resolutions.
/// </summary>
void ConfigureCanvasScaler()
{
canvasScaler.uiScaleMode = CanvasScaler.ScaleMode.ScaleWithScreenSize;
canvasScaler.referenceResolution = new Vector2(1920, 1080);
canvasScaler.screenMatchMode = CanvasScaler.ScreenMatchMode.MatchWidthOrHeight;
canvasScaler.matchWidthOrHeight = 0.5f;
}
/// <summary>
/// Adjusts the UI elements based on the screen size and resolution.
/// </summary>
void AdjustUIElements()
{
// Example: Adjust the size and position of a UI element
RectTransform uiElement = GetComponent<RectTransform>();
uiElement.sizeDelta = new Vector2(Screen.width * 0.1f, Screen.height * 0.1f);
uiElement.anchoredPosition = new Vector2(Screen.width * 0.5f, Screen.height * 0.5f);
}
}
Code language: HTML, XML (xml)
In this code example, we define a ScalableUI
class that configures the CanvasScaler
component to adapt to different screen sizes and resolutions. The ConfigureCanvasScaler
method sets the uiScaleMode
to ScaleWithScreenSize
and defines a reference resolution of 1920×1080. The screenMatchMode
is set to MatchWidthOrHeight
, and the matchWidthOrHeight
property is set to 0.5f, ensuring a balanced scaling between width and height.
The AdjustUIElements
method demonstrates how to adjust the size and position of a UI element based on the screen size and resolution. In this example, the size of the UI element is set to 10% of the screen width and height, and its position is centered on the screen.
By using these techniques, developers can create a scalable UI framework that adapts to different screen sizes and resolutions, ensuring a consistent user experience across all devices.
Future of Scalable UI Frameworks in Multiplayer Games
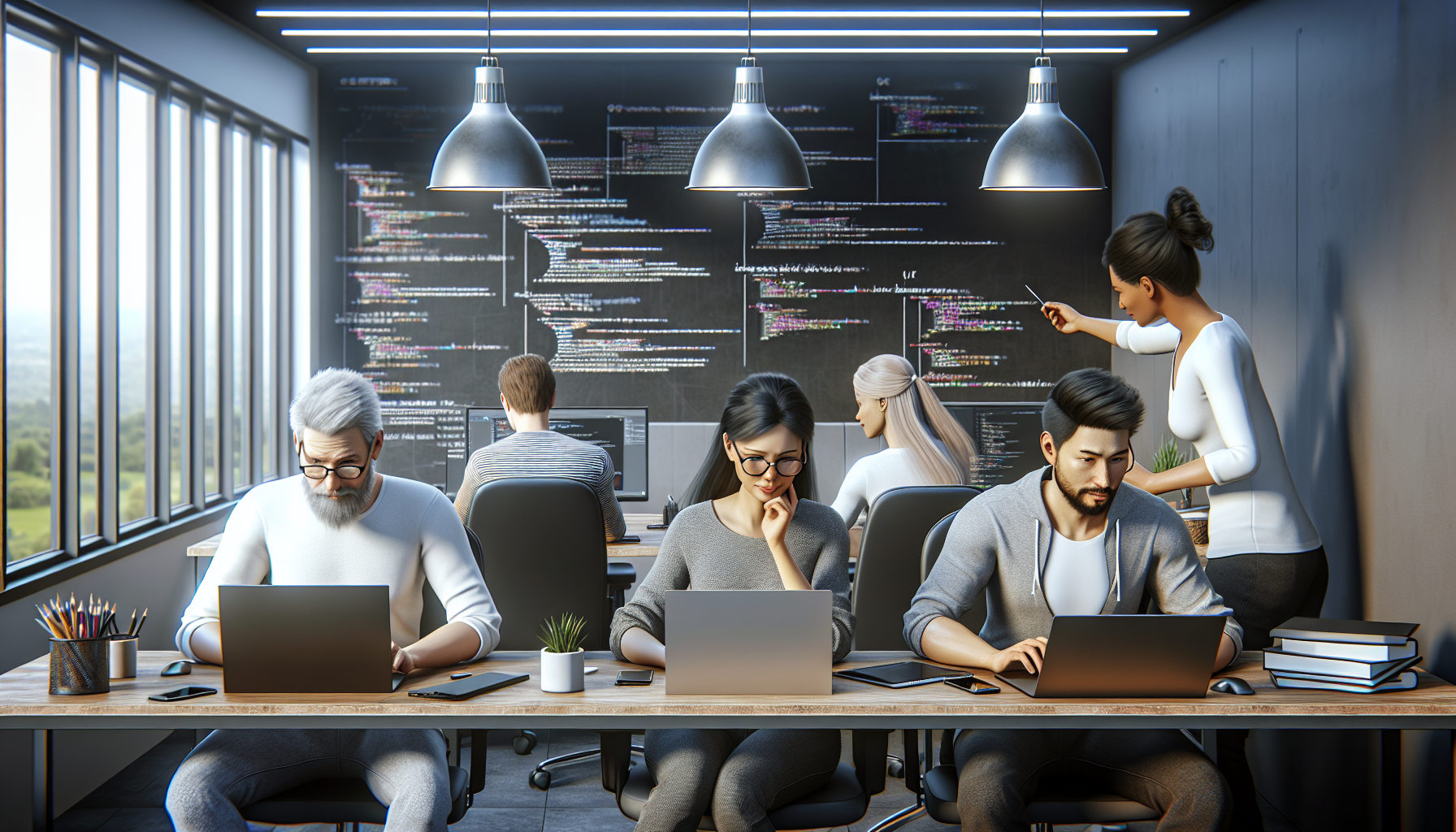
The future of scalable UI frameworks in multiplayer games is promising, with several trends and advancements shaping the industry. Here are five predictions for the future of scalable UI frameworks in multiplayer games:
- Increased Use of AI and Machine Learning: AI and machine learning will play a significant role in automating the design and optimization of scalable UI frameworks, making the development process more efficient and effective.
- Enhanced Cross-Platform Compatibility: As the gaming industry continues to evolve, there will be a greater emphasis on cross-platform compatibility, ensuring that scalable UI frameworks work seamlessly across different devices and operating systems.
- Improved Performance Optimization: Advances in hardware and software technologies will enable developers to create more efficient and optimized scalable UI frameworks, reducing the impact on game performance and enhancing the overall user experience.
- Greater Focus on Accessibility: There will be an increased focus on accessibility in scalable UI frameworks, ensuring that games are inclusive and accessible to players with disabilities, providing a more inclusive gaming experience.
- Integration of Augmented Reality (AR) and Virtual Reality (VR): The integration of AR and VR technologies will drive the development of new and innovative scalable UI frameworks, creating immersive and interactive gaming experiences for players.
Disclaimer
This is an AI-generated article with educative purposes and doesn’t intend to give advice or recommend its implementation. The goal is to inspire readers to research and delve deeper into the topics covered in the article.
- Remote Hiring in 2025 - April 5, 2025
- Burnout in Remote Teams: How It’s Draining Your Profits - January 27, 2025
- Signs You’re Understaffed - January 20, 2025