Understanding Game Security in Unreal Engine
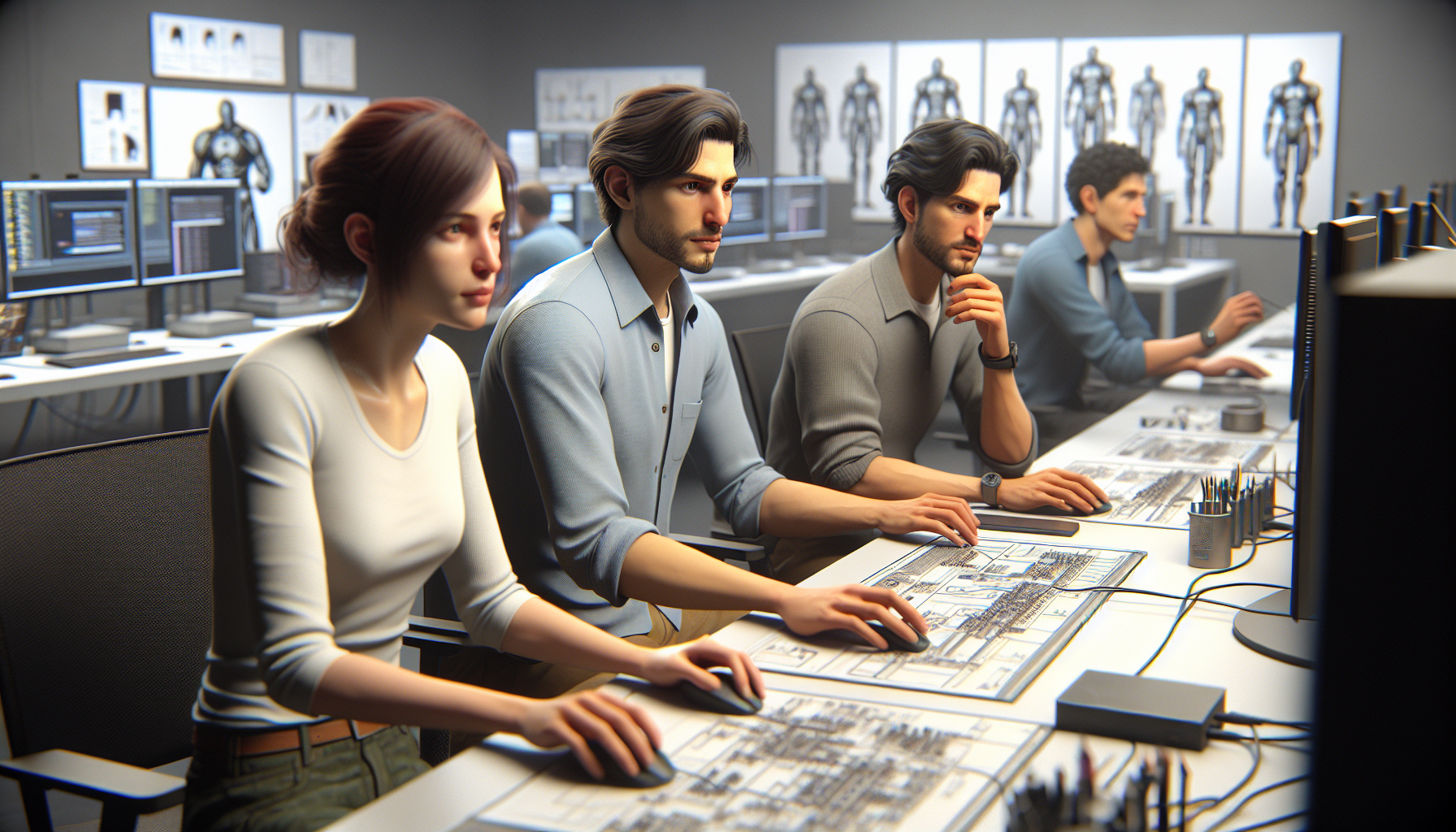
Key Points
- Even if players are hidden in a game, their location data is still known to all clients, which poses a security risk.
- Effective anti-cheat measures require server-side detection and mitigation rather than just prevention.
- Encrypting update packets can temporarily hinder cheating but is not a long-term solution.
- Advanced techniques like those used in VALORANT’s Fog of War system are effective but may not be feasible for all game types or indie developers.
- Understanding the balance between client-side and server-side updates is crucial for developing secure multiplayer games.
Basics of Game Security
Game security in multiplayer environments, especially within the Unreal Engine, revolves around ensuring fair play and preventing cheating. Cheating can range from exploiting game bugs to using sophisticated software that manipulates game data. The Unreal Engine provides various tools and features to help developers secure their games, but understanding and implementing these features effectively is crucial.
One fundamental aspect of game security is managing how data is shared between the server and clients. Unreal Engine uses a powerful networking model that supports efficient data replication and state synchronization across players. However, developers must carefully control what data is sent to each client to prevent potential exploits.
Challenges in Implementing Security Measures
Implementing robust security measures in multiplayer games can be challenging. Developers must anticipate and counteract a wide range of potential cheats and exploits. This requires a deep understanding of both the game’s mechanics and the underlying network and software architectures.
Moreover, the need to balance security with game performance and player experience adds another layer of complexity. Too stringent security measures might hinder game performance or overly complicate the gameplay, which could detract from the user experience. Developers must find an optimal balance that ensures both security and a smooth, engaging player experience.
The Problem of Data Exposure in Multiplayer Games
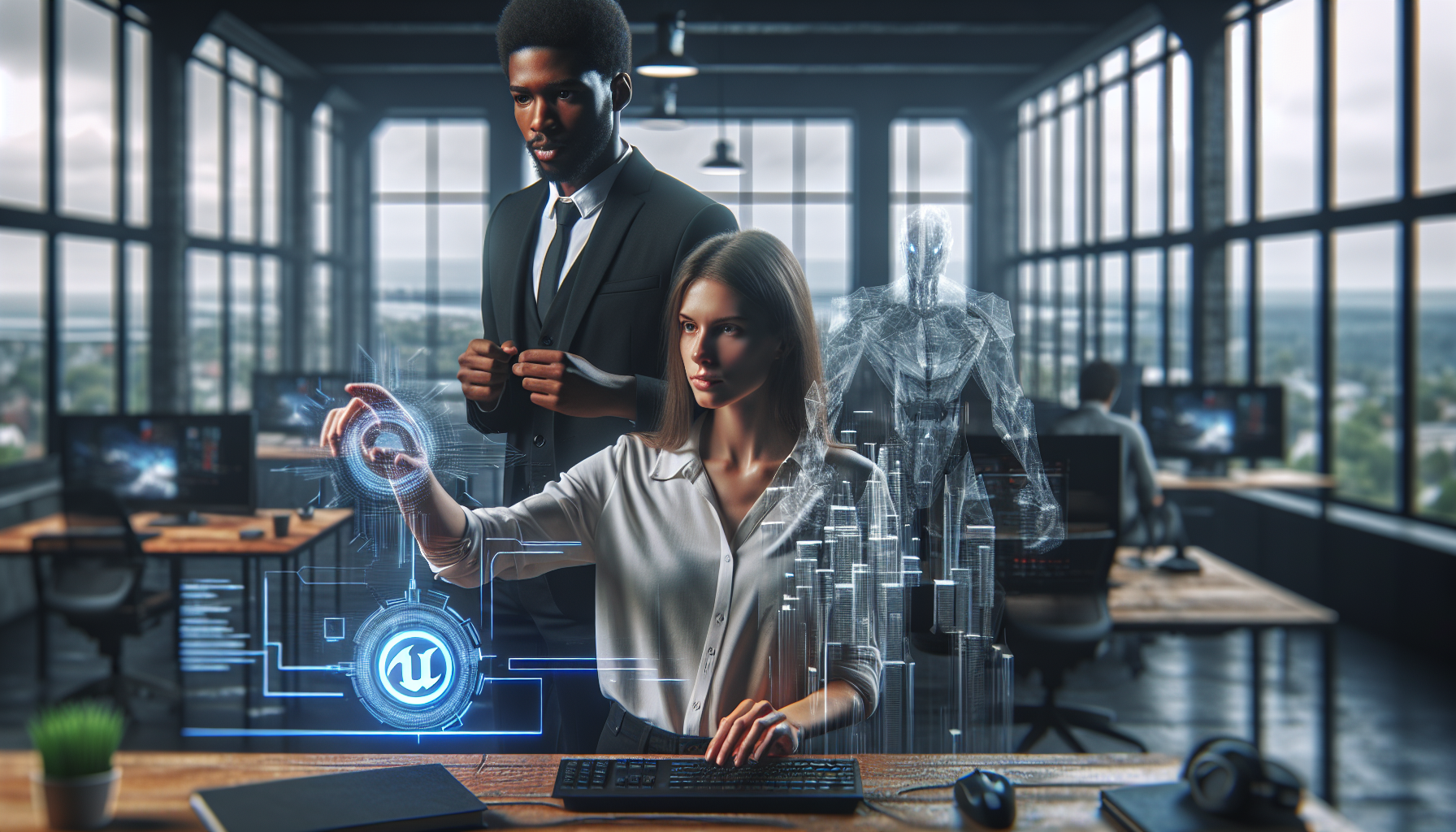
Understanding the Risk
In multiplayer games, particularly those built with Unreal Engine, a significant challenge is managing how much data each client has access to. If a client knows too much, it can lead to cheating, such as players being able to locate hidden enemies or foresee game events. This issue is often referred to as the “over-replication” of data.
The risk is particularly pronounced in games where players can gain a competitive advantage through knowledge of the environment or other players’ statuses. For example, in a tactical shooter, knowing an opponent’s location without having a direct line of sight can drastically alter the competitive balance of the game.
Impact on Game Integrity
When players exploit data exposure, it compromises the integrity of the game. This not only affects the fairness of the game but can also impact player retention and the overall success of the game. Players who feel that the competitive environment is compromised may be less likely to continue playing or investing in the game.
Furthermore, news of such exploits can quickly spread within the gaming community, potentially damaging the game’s reputation and dissuading new players from joining. Maintaining the integrity of the game is crucial for both its short-term popularity and long-term viability.
Case Studies and Examples
One notable example is the issue faced by PUBG, a popular game developed with Unreal Engine. The game struggled with cheaters who used radar hacks to view the positions of all players on the map. This was made possible because critical data about player locations was being sent to all clients, regardless of their actual in-game proximity or line of sight.
Another example is Riot Games‘ approach to their tactical shooter, VALORANT. They developed a sophisticated system known as “Fog of War” that meticulously controls what each client knows about other players’ positions, significantly reducing the opportunities for cheating through wallhacks.
Solutions to Prevent Data Exposure
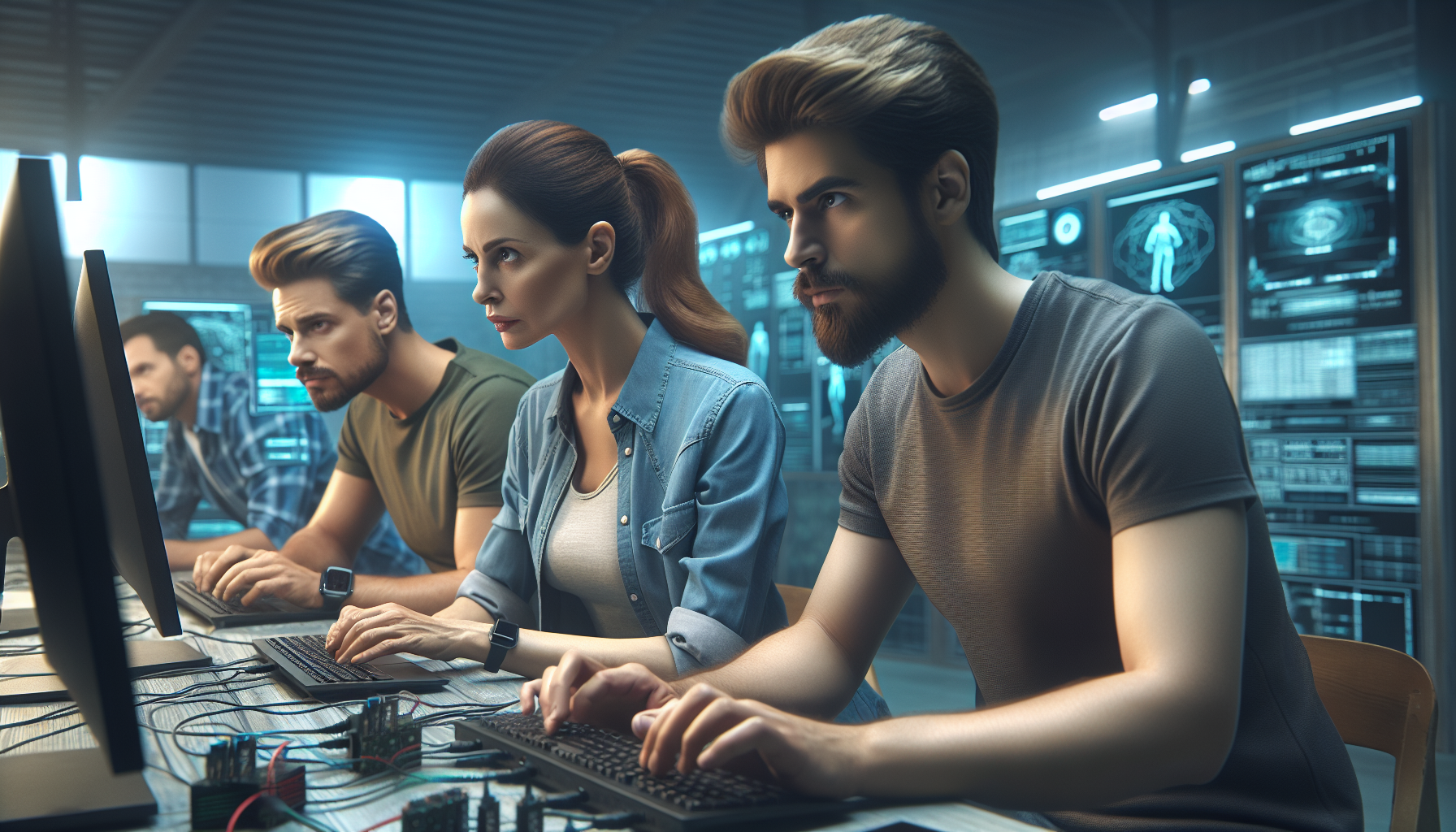
Step 1: Implementing Data Minimization
The first step in securing a multiplayer game in Unreal Engine is to implement data minimization. This involves ensuring that the server only sends necessary information to each client. For instance, if a player’s character is not visible to another player, their exact location should not be transmitted to that player’s client.
This can be achieved by enhancing the game’s logic to assess whether certain information is necessary for a client’s gameplay experience. Techniques such as interest management and relevance checks are critical in this process.
Step 2: Using Encryption and Secure Protocols
The second step involves encrypting the data sent between the server and clients and using secure communication protocols. This prevents unauthorized interception and manipulation of critical game data during transmission.
While encryption does not prevent cheating by itself, it adds a layer of security that makes it more difficult for cheaters to exploit data. It is particularly effective against man-in-the-middle attacks where data is intercepted during transmission.
Step 3: Continuous Monitoring and Updates
The final step is to set up systems for continuous monitoring of game activity to detect unusual patterns that may indicate cheating. This should be complemented by regular updates to the game’s security measures to address newly discovered vulnerabilities and cheating methods.
Continuous monitoring allows developers to react quickly to incidents of cheating and to update their security measures accordingly. This proactive approach is essential in maintaining the integrity of the game over time.
Code Example: Implementing Basic Anti-Cheat Logic in Unreal Engine
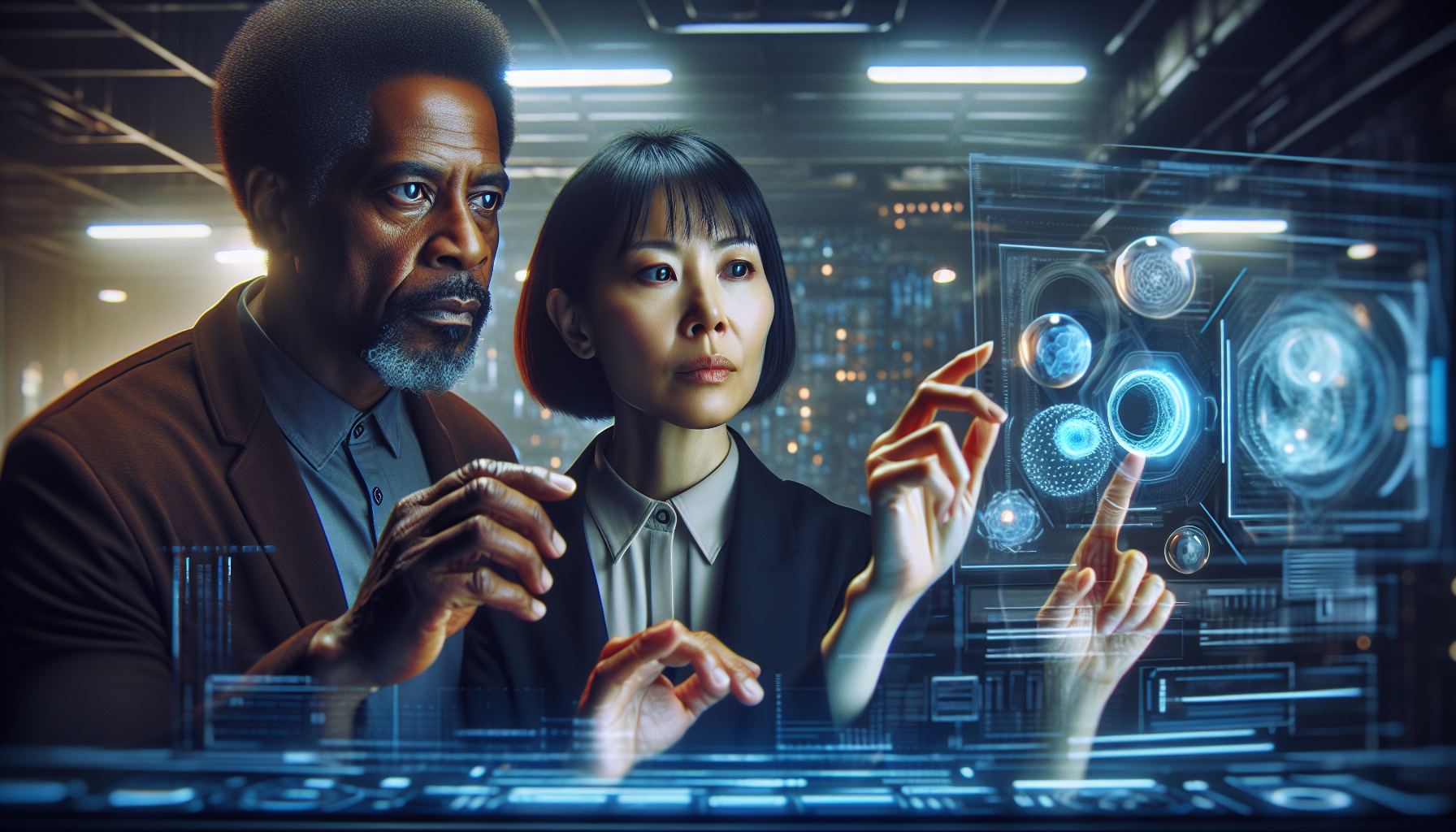
This section provides a practical example of how to implement basic anti-cheat logic in Unreal Engine. It focuses on minimizing data exposure to prevent cheating.
// Example of a basic anti-cheat mechanism in Unreal Engine
class AntiCheatController {
public:
// Constructor
AntiCheatController() {}
// Method to check player visibility and decide data transmission
bool ShouldTransmitData(Player* player, Player* target) {
// Check if the target player is visible to the player
if (!IsPlayerVisible(player, target)) {
return false; // Do not transmit data if target is not visible
}
return true; // Transmit data if target is visible
}
private:
// Method to determine if a player is visible to another player
bool IsPlayerVisible(Player* player, Player* target) {
// Implement visibility logic here
// This could involve checking line of sight, occlusion, etc.
return true; // Simplified for example purposes
}
};
Code language: C# (cs)
The code above defines a class AntiCheatController
that includes methods to determine whether data about a player should be transmitted based on their visibility to another player.
This is a fundamental aspect of preventing cheating by controlling data exposure.
Frequently Asked Questions
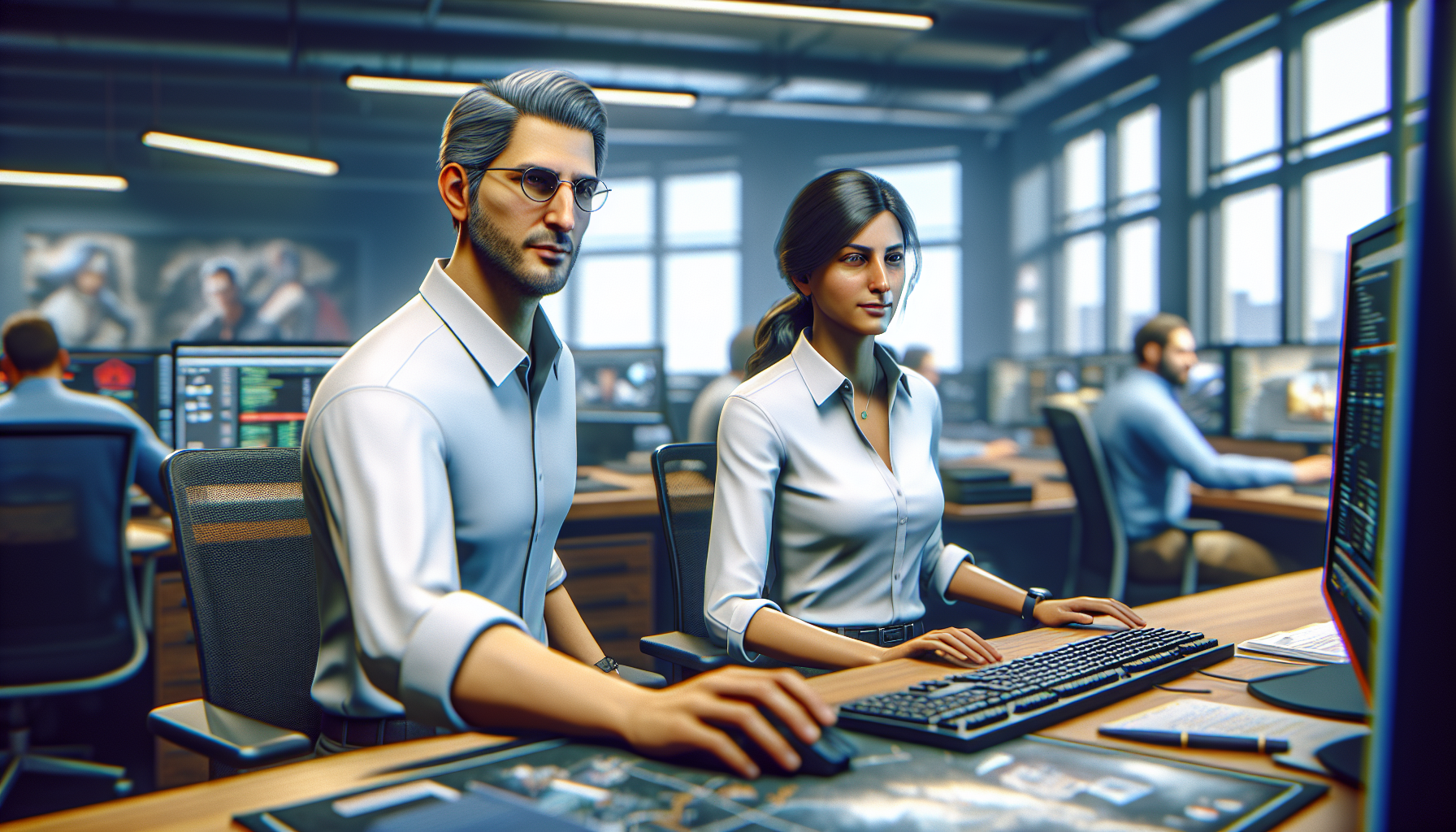
How can Unreal Engine help in combating cheating in multiplayer games?
Unreal Engine provides robust networking capabilities and a powerful scripting environment that allows developers to implement sophisticated security measures. These include controlling data replication, implementing server-authoritative logic, and using built-in features to monitor and adjust gameplay based on player behavior.
Additionally, Unreal Engine’s active developer community and comprehensive documentation provide valuable resources for learning about and implementing anti-cheat measures.
What are some common types of cheats in multiplayer games?
Common cheats include wallhacks (seeing through walls), aimbots (automated aiming), and speed hacks (increasing movement speed). Cheaters may also exploit game bugs or manipulate game data sent between the client and server to gain an unfair advantage.
Addressing these cheats requires a combination of client-side and server-side checks, data encryption, and continuous monitoring of game activity for unusual patterns.
Is it possible to completely eliminate cheating in multiplayer games?
While it is extremely difficult to completely eliminate cheating, effective security measures can significantly reduce its incidence and impact. A multi-layered approach that includes technical solutions, regular updates, and community management is most effective.
Developers must stay informed about the latest cheating techniques and continuously evolve their security strategies to address new threats.
Can indie developers effectively implement game security measures?
Yes, indie developers can implement effective security measures, though they may face resource constraints. Leveraging built-in Unreal Engine features, prioritizing key security areas, and engaging with the community for support and ideas can help indie developers protect their games against cheating.
Open-source tools and community-shared knowledge also provide accessible options for enhancing game security without substantial investment.
Looking Ahead: The Future of Game Security
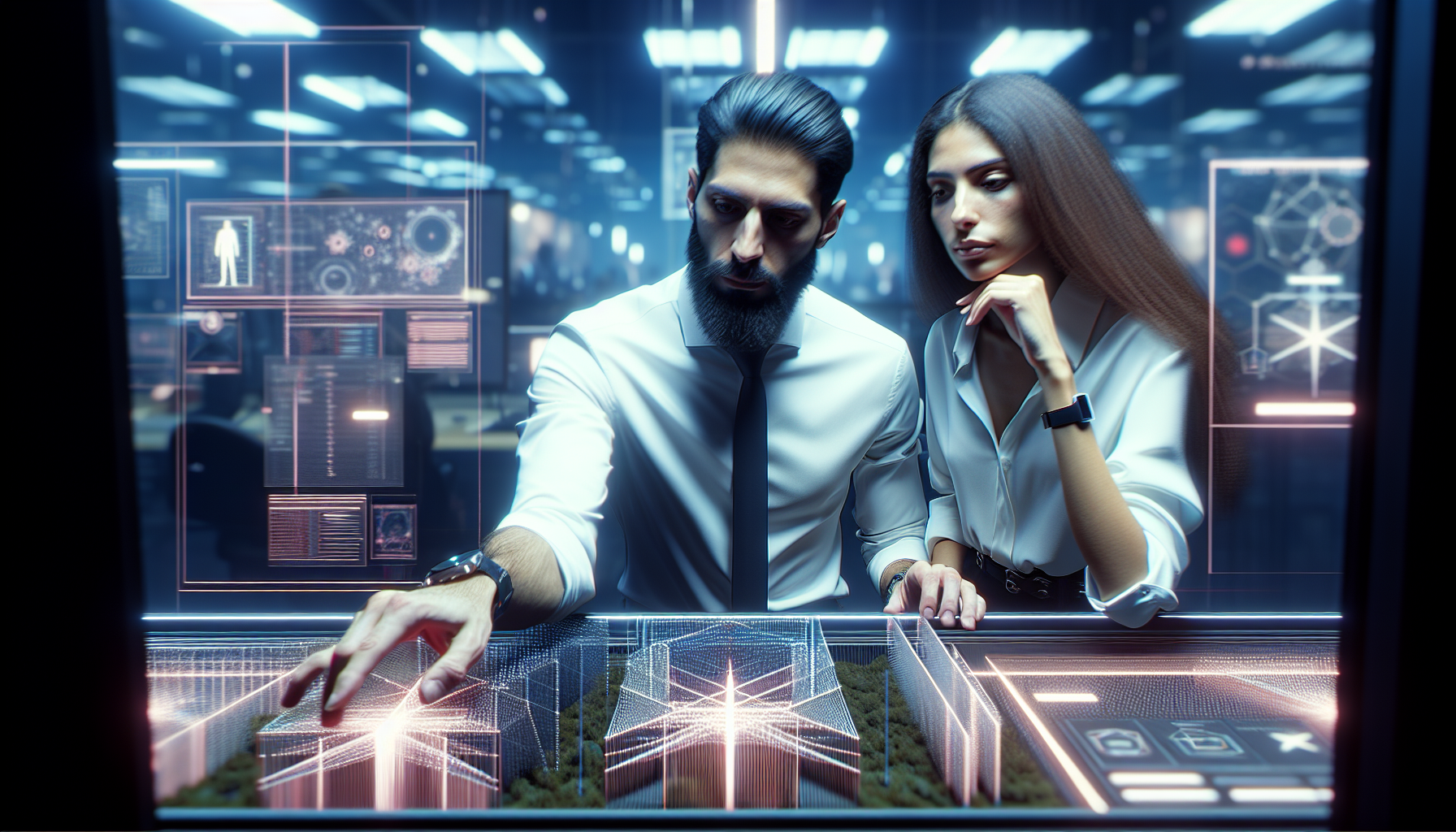
As technology advances, so too do the methods used by cheaters, making ongoing innovation in game security essential. Here are five predictions for the future of game security:
- Increased use of machine learning: Machine learning algorithms will become more prevalent in detecting unusual player behavior and identifying potential cheats.
- Enhanced player verification processes: Multi-factor authentication and other verification methods will become standard to verify player identities and reduce fraud.
- Greater reliance on cloud-based security: More games will operate on cloud platforms that offer advanced security protocols and real-time cheat detection.
- Development of standardized anti-cheat software: There will be a move towards standardized anti-cheat solutions that can be easily implemented by developers of all sizes.
- Increased legal actions: There will be more legal actions against cheat developers and distributors, which will deter the development and distribution of cheating tools.
More Information
- Official Unreal Engine Website
- Epic Developer Community Forums
- Epic Games Acquires Kamu Game Security
- Reddit Discussion on Making a Game Like FNaF in UE4
Disclaimer
This article is AI-generated with educational purposes in mind and does not intend to provide specific advice or recommend its implementation. It aims to inspire further research and exploration into the topics covered.
- What challenges do companies face in detecting AI-generated content? - May 17, 2024
- What Skills Are Necessary for Product Managers to Effectively Implement AI Content Strategies? - May 17, 2024
- How does deep learning AI differ from traditional machine learning regarding security applications? - May 17, 2024