Overview of Accessibility in Unity Game UIs
Key Points
- Utilize the UI Accessibility Plugin (UAP) from MetalPop Games to enhance user interface accessibility.
- Explore additional GUI tools available on the Unity Asset Store.
Importance of Accessibility in Gaming
Accessibility in gaming ensures that all players, regardless of their physical or cognitive abilities, can enjoy the game. This inclusivity not only broadens the market reach for developers but also promotes equality and diversity within the gaming community. Implementing strong accessibility features in game UIs allows players with disabilities to experience games more fully, contributing to higher satisfaction and engagement levels.
Unity is one of the leading game development platforms. It provides various tools and plugins to enhance accessibility. The Accessibility page on Unity’s website offers insights and resources that help developers understand and implement accessibility features effectively.
Moreover, the UI Accessibility Plugin (UAP) available on the Unity Asset Store is a popular choice among developers looking to improve their game’s UI accessibility. This tool simplifies the integration of accessibility features, such as screen readers and subtitle options, making it easier for developers to comply with accessibility standards.
Challenges in Implementing Accessibility
Despite the availability of tools and resources, implementing accessibility in game UIs presents several challenges. One major challenge is the lack of awareness and understanding of accessibility needs among game developers. This can lead to oversights in design, which may alienate players with disabilities.
Another challenge is the technical difficulty of retrofitting existing games with accessibility features. This often requires significant changes to the game’s codebase and UI, which can be time-consuming and costly. Additionally, testing for accessibility issues requires specialized knowledge and tools, which might not be readily available in all development environments.
Lastly, there is the challenge of balancing game design with accessibility. Developers must ensure that adding accessibility features does not alter the core gameplay, which can be particularly tricky in games where timing and precision are key elements.
The Challenge of Inclusive Design in Game Development
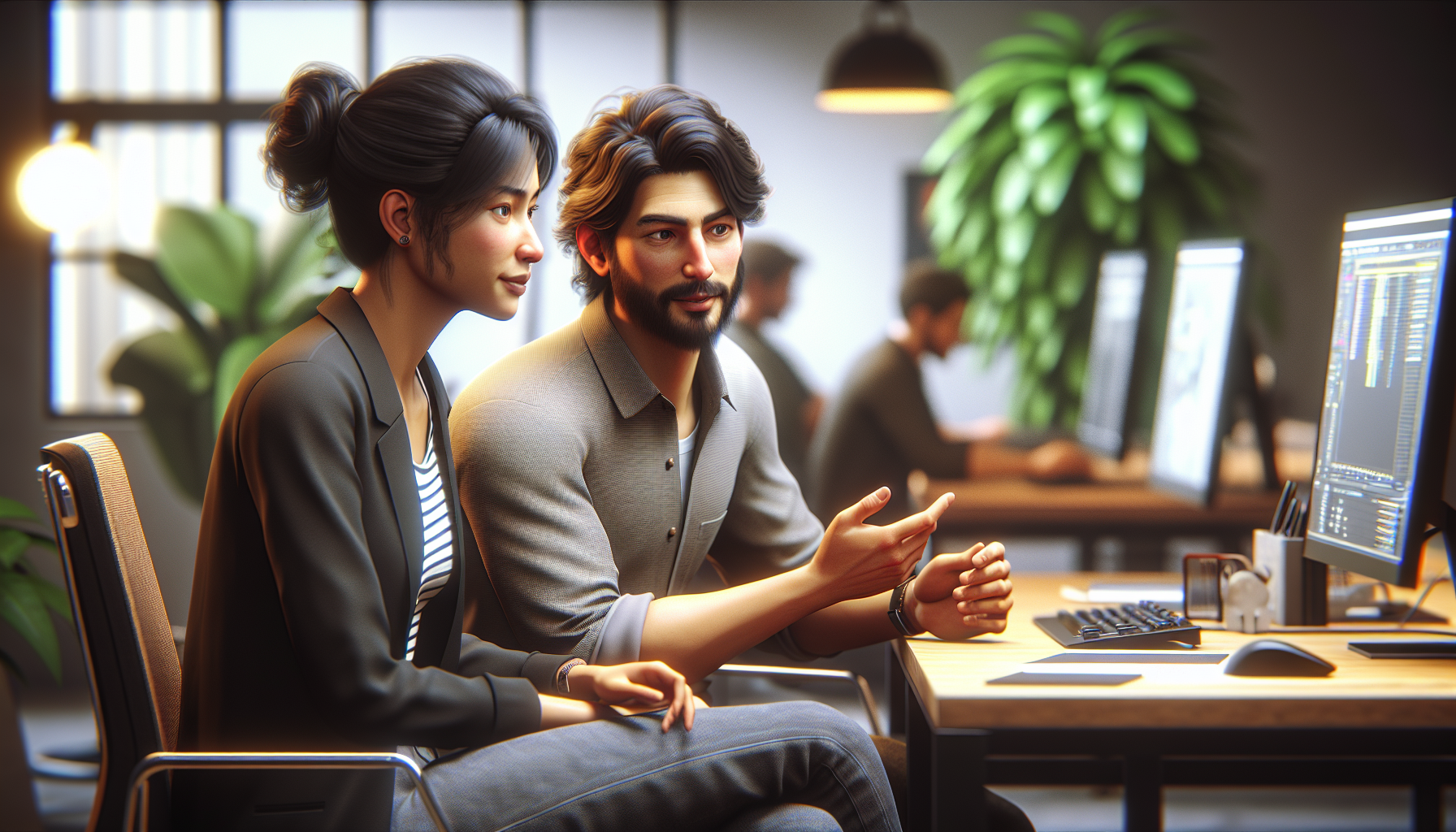
Understanding the Scope
In game development, creating an inclusive design that caters to all players, including those with disabilities, is a formidable challenge. This involves more than just adding subtitles or colorblind modes; it requires a deep integration of accessibility into the game’s design from the outset.
For instance, many games rely heavily on visual and auditory cues, which can be a barrier for players with visual or hearing impairments. The challenge is to provide these players with alternative ways to receive these cues without disrupting the gameplay experience for others.
Technical and Resource Constraints
Small to medium-sized game studios often face significant technical and resource constraints, making it difficult to prioritize and implement comprehensive accessibility features. These limitations can stem from restricted budgets, limited staff expertise, or the pressure to meet tight release schedules.
Moreover, the lack of standardized guidelines for game accessibility poses an additional hurdle. Developers must often rely on a patchwork of resources and tools, such as the UI Accessibility Plugin (UAP), to understand and implement accessibility standards, which can lead to inconsistent user experiences.
Impact on Player Base
Failing to incorporate accessibility features can have a direct impact on a game’s player base. Games that are not accessible may exclude a significant portion of the population that experiences some form of disability. This not only limits the game’s market reach but can also lead to negative publicity and player feedback, affecting the game’s success and the studio’s reputation.
Conversely, games that are known for their high level of accessibility can attract a wider audience, including advocacy groups and players who value inclusivity. This can lead to positive word-of-mouth, higher sales, and a more dedicated player community.
Solving Accessibility Challenges in Unity Game UIs
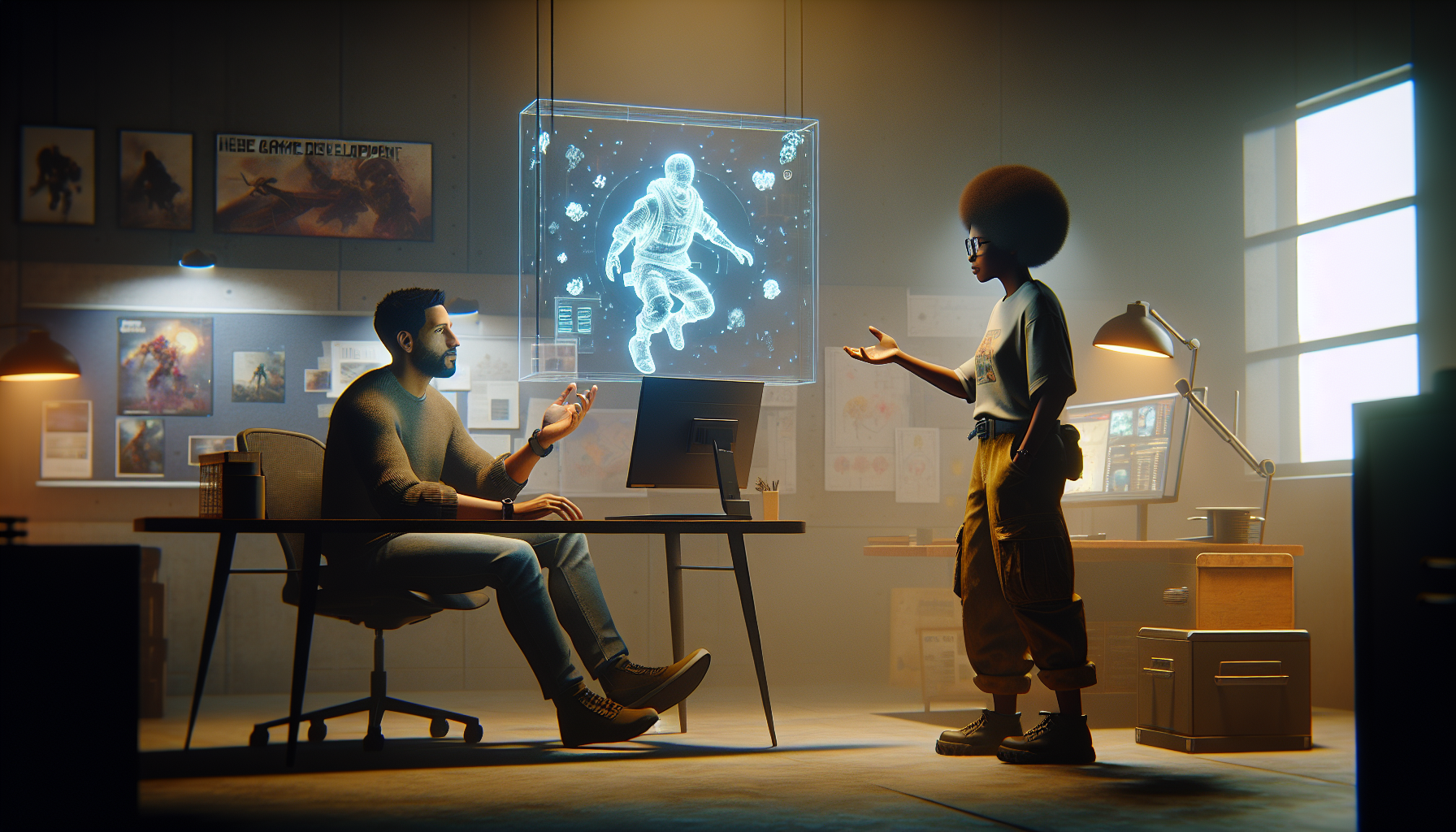
Step 1: Conducting an Accessibility Audit
The first step in enhancing accessibility in Unity game UIs is to conduct a thorough accessibility audit. This involves reviewing the current game design and identifying elements that are not accessible to players with disabilities. Tools like the UI Accessibility Plugin (UAP) can be instrumental in this process, offering automated checks and suggestions for improvements.
During the audit, focus on key areas such as visual elements, audio cues, control schemes, and interface navigation. It’s important to involve players with disabilities in this process, as their firsthand experience can provide valuable insights that automated tools might miss.
Step 2: Implementing UI Adjustments
Based on the findings from the accessibility audit, the next step is to implement UI adjustments. This might include adding subtitle options, colorblind modes, or customizable control schemes. Unity’s flexible UI system allows for these adjustments to be made relatively easily, with support from plugins and community resources.
It’s crucial to ensure that these adjustments are tested extensively to avoid introducing new usability issues. Regular updates and patches may be necessary to refine the accessibility features based on player feedback and ongoing testing.
Step 3: Continuous Improvement and Community Engagement
The final step is to establish a process for continuous improvement and community engagement. Accessibility should be an ongoing commitment, not a one-time effort. By keeping lines of communication open with players, especially those with disabilities, developers can continue to learn and improve the game’s accessibility.
Engaging with the community can also help in identifying emerging accessibility tools and practices. Participating in forums, attending conferences, and collaborating with accessibility advocates can provide new ideas and insights that enhance the game’s inclusivity.
Code Example: Implementing an Accessible Menu in Unity
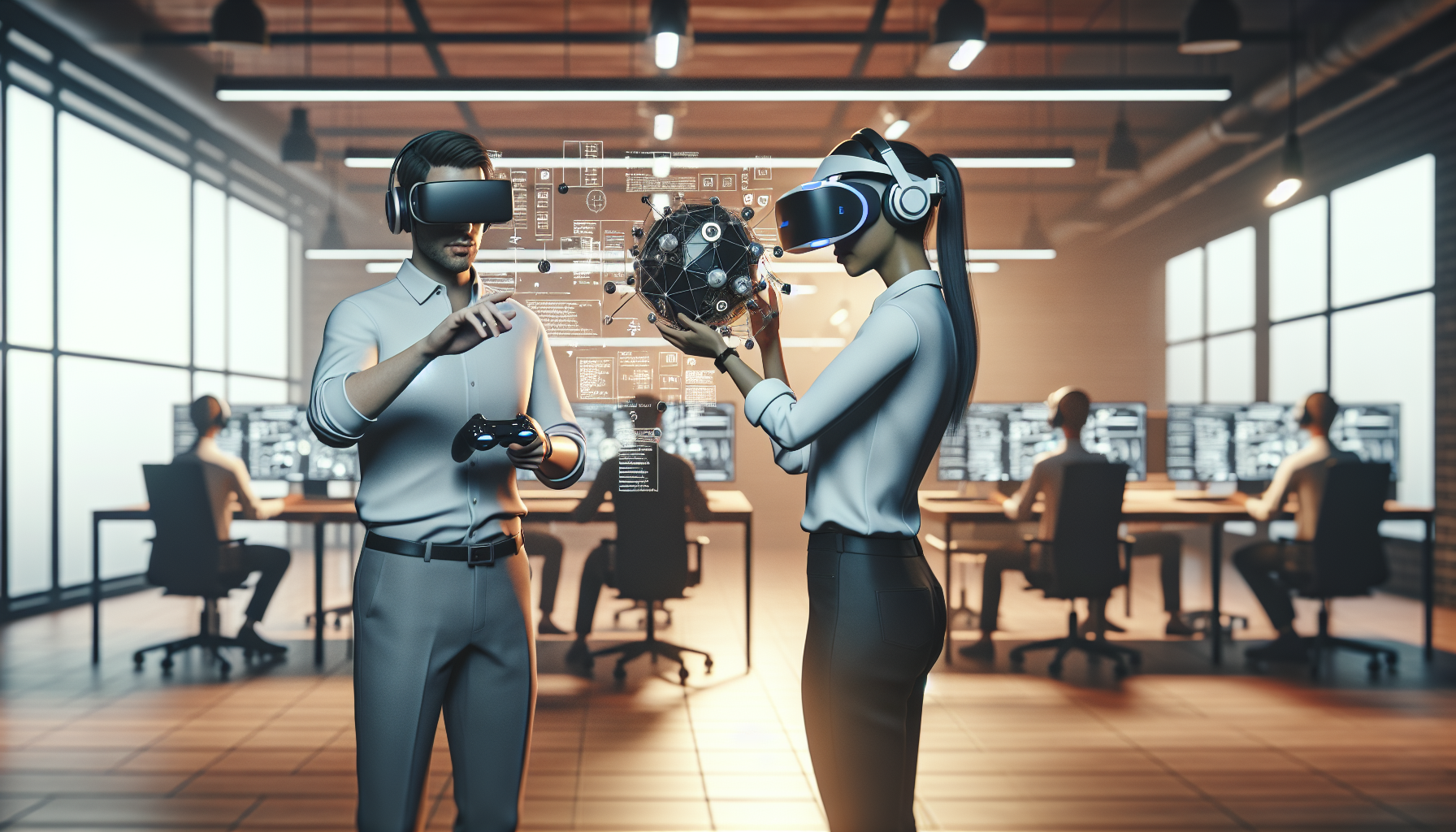
This section provides a practical example of how to implement an accessible menu in Unity using C#. The example includes a simple menu system with accessibility features such as high contrast mode and screen reader support.
// AccessibleMenu.cs
using UnityEngine;
using UnityEngine.UI;
public class AccessibleMenu : MonoBehaviour
{
public Text menuText;
public Button[] menuButtons;
private int selectedIndex = 0;
void Start()
{
UpdateMenu();
}
void Update()
{
if (Input.GetKeyDown(KeyCode.UpArrow))
{
selectedIndex = Mathf.Max(selectedIndex - 1, 0);
UpdateMenu();
}
else if (Input.GetKeyDown(KeyCode.DownArrow))
{
selectedIndex = Mathf.Min(selectedIndex + 1, menuButtons.Length - 1);
UpdateMenu();
}
}
private void UpdateMenu()
{
for (int i = 0; i < menuButtons.Length; i++)
{
menuButtons[i].GetComponent().color = (i == selectedIndex) ? Color.yellow : Color.white;
}
menuText.text = "Use arrow keys to navigate the menu. Press Enter to select " + menuButtons[selectedIndex].name + ".";
}
}
Code language: C# (cs)
The code above defines a simple menu system where the user can navigate through different options using the arrow keys.
The selected option is highlighted in yellow, and instructions for navigation are displayed on the screen.
This example can be expanded with additional features such as audio feedback and alternative control schemes to enhance accessibility further.
Frequently Asked Questions
How can I test the accessibility of my Unity game?
To test the accessibility of your Unity game, start by using automated tools like the UI Accessibility Plugin (UAP), which can help identify common accessibility issues. However, the most effective method is to conduct user testing with players who have disabilities. This direct feedback can provide insights that automated tools cannot.
Additionally, consulting accessibility guidelines such as the Web Content Accessibility Guidelines (WCAG) and the Game Accessibility Guidelines should be considered. These resources offer valuable information on creating accessible digital content and can be adapted to the context of game development.
What are some common accessibility features in games?
Common accessibility features in games include subtitles and closed captions, colorblind modes, control remapping, and difficulty adjustments. More advanced features might include full keyboard and mouse support, voice control, and compatibility with assistive technologies like screen readers.
Implementing these features involves both design and technical challenges, but the benefits of reaching a wider audience and improving the gaming experience for all players are substantial. Tools and resources available through platforms like Unity can help streamline this process.
Can accessibility improvements affect the gameplay experience for other players?
When properly implemented, accessibility improvements should not negatively affect other players’ gameplay experience. In fact, many accessibility features, such as the ability to remap controls or adjust text sizes, can benefit all players by allowing for a more personalized and comfortable gaming experience.
It’s important to design these features so that they can be toggled on or off according to the player’s preferences. This approach ensures that the game remains enjoyable for everyone, regardless of their individual needs or abilities.
Are there legal requirements for accessibility in games?
In some regions, legal requirements for accessibility in digital products, including games, exist. For example, in the United States, the Americans with Disabilities Act (ADA) and the 21st Century Communications and Video Accessibility Act (CVAA) set forth guidelines that may apply to game developers.
It’s advisable to consult with legal experts to understand the specific requirements in your region. Additionally, adhering to widely recognized accessibility standards, even when not legally required, can help ensure that your game is accessible to as many players as possible.
Speculating on the Future of Game Accessibility
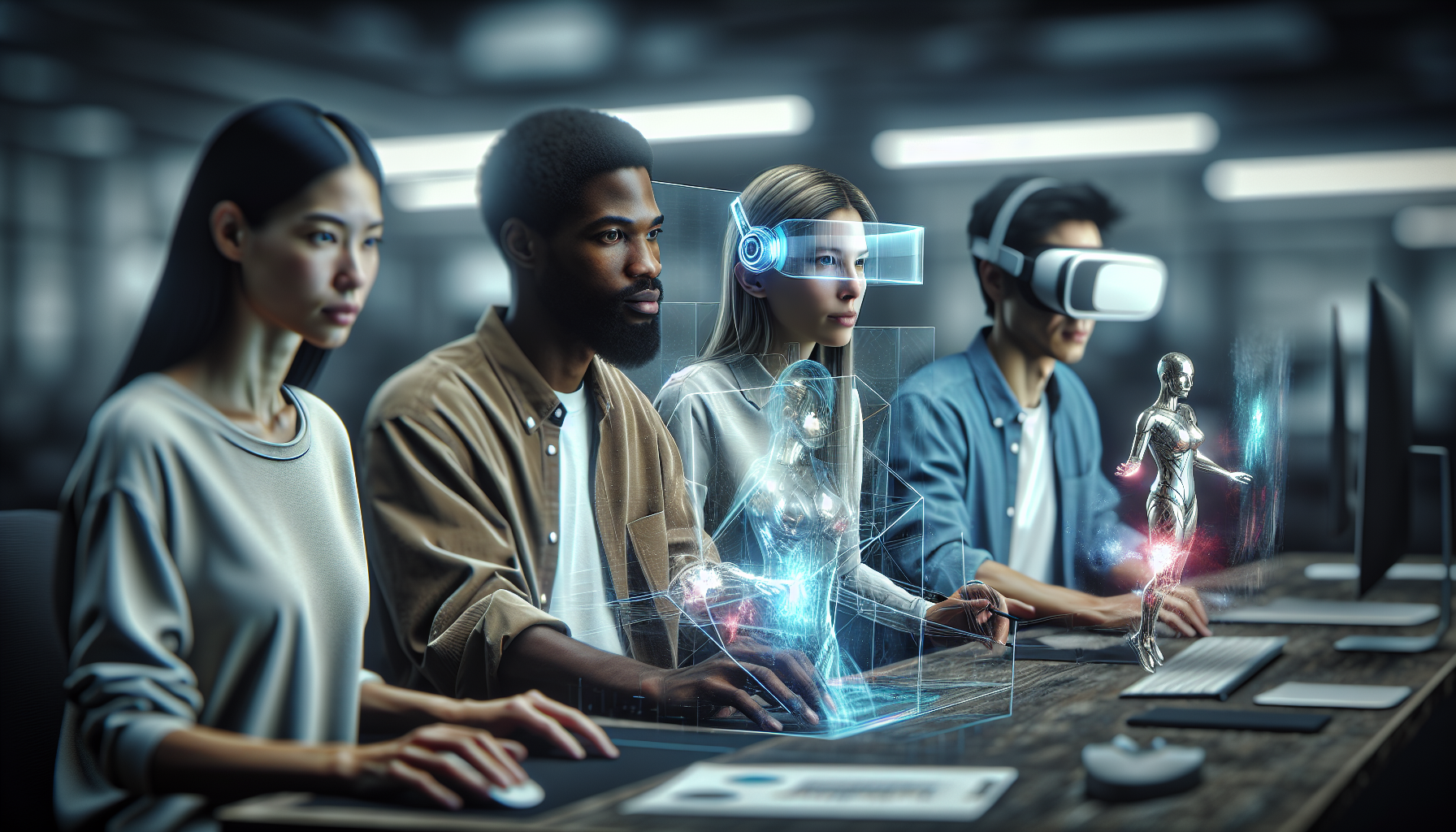
As technology advances and awareness of inclusivity grows, the future of game accessibility looks promising. Here are five predictions for the coming years:
- Increased Regulatory Pressure: Governments worldwide may introduce more stringent regulations requiring video games to include comprehensive accessibility features similar to those for other digital services.
- Advancements in Assistive Technologies: New technologies, such as improved voice recognition and AI-driven adaptive interfaces, could revolutionize how players with disabilities interact with games.
- Greater Community Involvement: Game development communities are likely to become more proactive in promoting accessibility, with more resources and events dedicated to this cause.
- Enhanced Developer Tools: Game development platforms like Unity might offer more advanced and integrated accessibility tools, making it easier for developers to implement these features.
- Broader Industry Adoption: As awareness of the importance of accessibility grows, more game developers and publishers will prioritize inclusivity, leading to a broader adoption of accessibility standards across the industry.
More Information
- Unity Accessibility Fundamentals – The official Unity page provides resources and guidelines for implementing accessibility in games.
- UI Accessibility Plugin (UAP) – A tool available on the Unity Asset Store that helps developers integrate accessibility features into their games.
Disclaimer
This article is generated by AI for educational purposes and does not intend to provide specific advice or recommend its implementation. The content aims to inspire further research and exploration into the topics covered. Readers are encouraged to consult professional sources and experts before making any significant decisions based on the information provided.
- What challenges do companies face in detecting AI-generated content? - May 17, 2024
- What Skills Are Necessary for Product Managers to Effectively Implement AI Content Strategies? - May 17, 2024
- How does deep learning AI differ from traditional machine learning regarding security applications? - May 17, 2024