Overview of Emotion Detection in Gaming
Key Points
- Emotion recognition technology is rapidly advancing but faces significant scientific skepticism regarding its accuracy and ethical implications.
- Games that adapt to players’ emotions can potentially enhance engagement and personalization.
- Current technologies often misinterpret facial expressions, leading to incorrect emotion assessments.
- There is a growing concern about the privacy and ethical use of emotion detection technologies in games.
- Developers are exploring alternative methods for more accurate and less invasive emotion recognition.
What is Emotion Detection?
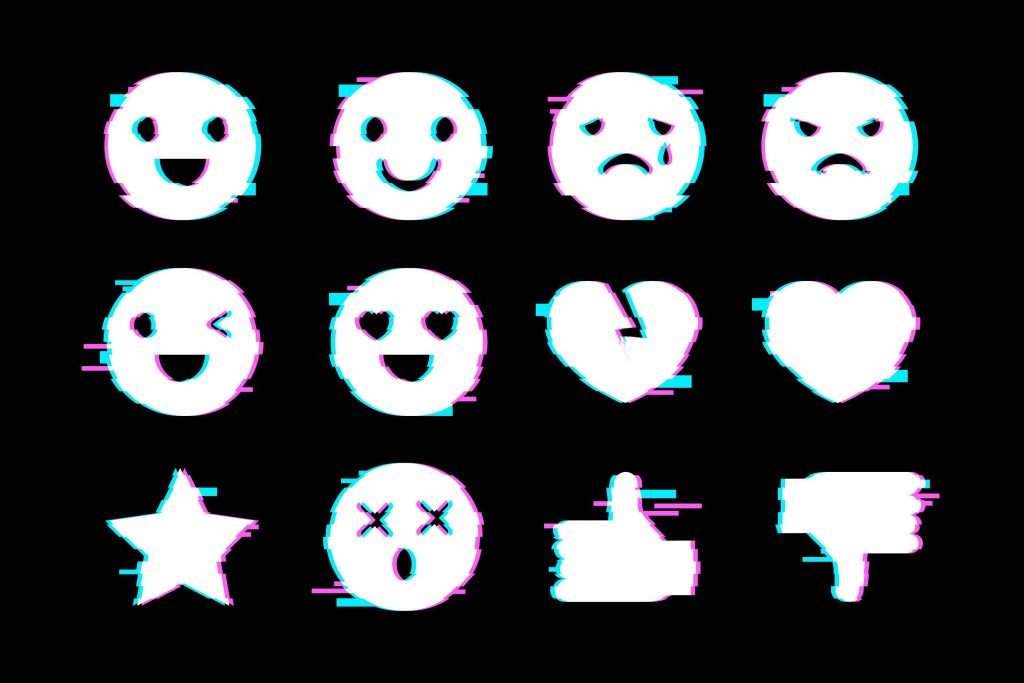
Emotion detection technology aims to identify human emotions using various data inputs, primarily focusing on facial expressions, voice intonations, and body language. In the context of video games, developers can use this technology to create more immersive and responsive gaming experiences by adjusting game dynamics based on the player’s emotional state.
However, the effectiveness of emotion detection relies heavily on the accuracy of the technology used. Current methods include machine learning algorithms that analyze video data from players’ webcams or sensors that monitor physiological responses. Despite advancements, the challenge remains in accurately interpreting subtle emotional cues that are often context-dependent.
Moreover, we cannot overlook the ethical considerations of such technologies. Privacy concerns arise when they collect sensitive emotional data, potentially exposing players to data breaches or unwanted surveillance. Thus, developers must navigate these challenges carefully to harness the benefits of emotion detection responsibly.
Applications in Video Games
Emotion detection can transform gaming experiences by allowing developers to tailor content in real time. For instance, if the system detects that the player is not sufficiently frightened, a horror game might intensify its scare factors. Conversely, a learning game could adapt its difficulty based on the player’s frustration levels to maintain an optimal challenge.
Another application is in narrative-driven games, where character interactions can change based on the player’s emotional responses, leading to dynamic storylines. This not only increases engagement but also adds a layer of personalization to the gaming experience, as each player might encounter a slightly different story based on their emotional reactions during gameplay.
Despite these potential benefits, developers must handle the implementation of emotion detection with care to avoid creating intrusive gameplay experiences and to ensure that they respect players’ privacy.
Challenges in Emotion Detection for Game Development
Accuracy and Reliability Issues
One of the primary challenges in implementing emotion detection gaming technology is ensuring its accuracy and reliability. Misinterpretations of emotional data can lead to gameplay adjustments that detract from the user experience rather than enhancing it.
For example, if a game mistakenly interprets a player’s excitement as anger, it could unnecessarily modify the game’s difficulty or narrative elements, leading to frustration.
Moreover, the variability in individual expressions of emotion poses a significant challenge. What one person expresses as joy, another might show as subdued contentment. This diversity means that a one-size-fits-all approach to emotion detection can end up being less effective, requiring more sophisticated, personalized calibration to function accurately across different players.
Integration with Existing Game Design
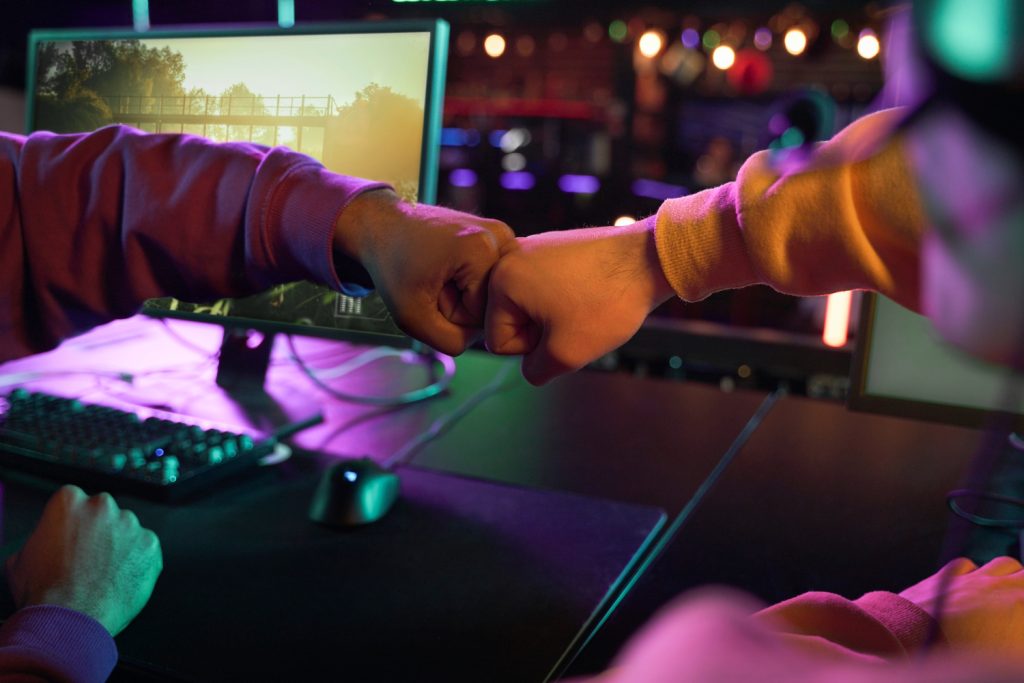
Integrating emotion detection technology into existing game designs presents practical challenges. It requires not only the seamless incorporation of new technology into established systems but also adjustments to game design principles to take full advantage of emotional data.
Game developers must consider how changes driven by emotion detection will affect gameplay mechanics, narrative flow, and overall player engagement. They must thoughtfully plan this integration to enhance the gaming experience without overwhelming or alienating players.
Solutions to Enhance Emotion Detection in Gaming
Step 1: Improved Data Collection Techniques
To address accuracy issues in emotion detection, developers can implement more advanced data collection techniques that combine multiple sources of information. For example, using both facial recognition software and biometric sensors can provide a more comprehensive understanding of a player’s emotional state.
Developers can calibrate these technologies to account for individual differences in emotional expression, potentially through initial calibration phases where the system learns how each player tends to express emotions during gameplay.
Step 2: Transparent Data Usage Policies
Developing clear and transparent data usage policies is crucial for addressing privacy concerns associated with emotion detection. Players should have full information about what data is collected, how it is used, and who has access to it. Moreover, giving players control over their data, such as options to opt out of data collection, can help build trust and encourage acceptance of emotion detection technologies.
These policies should also comply with relevant data protection laws, such as the General Data Protection Regulation (GDPR) in the European Union, to ensure that players’ data rights are respected and protected.
Step 3: Ethical Game Design Practices
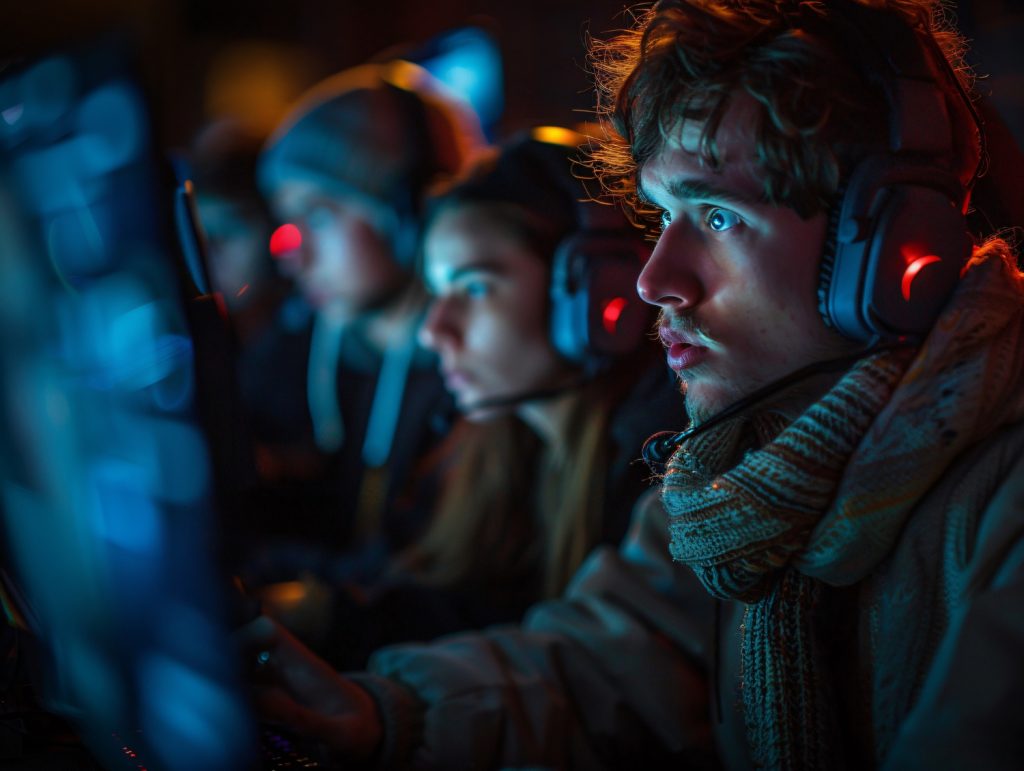
Implementing ethical game design practices is essential when integrating emotion detection technologies. This involves designing games in a way that respects player autonomy and avoids manipulative practices. For example, developers should not use emotional data to lead players into making in-game purchases or decisions that they might not have made otherwise.
Game developers should also consider the potential psychological impacts of their designs, ensuring that games using emotion detection do not cause undue stress or negative emotional experiences for players.
This ethical approach can help ensure that the use of emotion detection technology enhances the gaming experience positively and respectfully.
Code Example: Implementing Emotion Detection in a Game
This section provides a Python code example that demonstrates how to implement basic emotion detection in a game using the OpenCV and TensorFlow libraries. The example focuses on detecting facial expressions from webcam input and adjusting game elements based on the detected emotions.
import cv2
import tensorflow as tf
from tensorflow.keras.models import load_model
class EmotionDetector:
def __init__(self):
self.model = load_model('emotion_detection_model.h5')
self.face_cascade = cv2.CascadeClassifier('haarcascade_frontalface_default.xml')
def detect_emotions(self, frame):
"""
Detects faces in the frame and classifies each face's emotion.
"""
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
faces = self.face_cascade.detectMultiScale(gray, 1.3, 5)
for (x, y, w, h) in faces:
face = gray[y:y+h, x:x+w]
face = cv2.resize(face, (48, 48))
face = face.reshape(1, 48, 48, 1)
prediction = self.model.predict(face)
emotion = self.interpret_emotion(prediction)
cv2.rectangle(frame, (x, y), (x+w, y+h), (255, 0, 0), 2)
cv2.putText(frame, emotion, (x, y-10), cv2.FONT_HERSHEY_SIMPLEX, 0.9, (36,255,12), 2)
return frame
def interpret_emotion(self, prediction):
"""
Converts the model's prediction to a human-readable emotion.
"""
emotions = ['Angry', 'Disgust', 'Fear', 'Happy', 'Sad', 'Surprise', 'Neutral']
return emotions[np.argmax(prediction)]
# Main game loop
cap = cv2.VideoCapture(0)
detector = EmotionDetector()
while True:
ret, frame = cap.read()
if not ret:
break
frame = detector.detect_emotions(frame)
cv2.imshow('Emotion Detector', frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
The code initializes an instance of the EmotionDetector class, which uses a pre-trained deep-learning model to classify emotions based on facial expressions captured through a webcam. The detect_emotions method applies face detection to each frame of the webcam feed, and the interpret_emotion method translates the model’s predictions into human-readable emotions.
This setup enables real-time emotion detection that developers can integrate into game logic to modify gameplay based on the player’s emotional state.
FAQs on Emotion Detection in Gaming
How accurate is emotion detection technology in games?
Emotion detection technology in games is continually improving, but it still faces challenges in accuracy due to the complexity of human emotions and their expression. Factors such as individual differences in emotional expression and contextual nuances make it difficult for AI systems to achieve perfect accuracy. However, advancements in machine learning and data collection techniques are helping to improve the reliability of these systems.
Game developers need to ensure that the technology complements, rather than replaces, traditional gameplay elements to help mitigate the impact of any inaccuracies.
What are the ethical considerations for using emotion detection in games?
The use of emotion detection technology in games raises several ethical considerations, primarily concerning player privacy and data security. Collecting emotional data can be seen as intrusive, and without proper safeguards, it could lead to misuse or unintended consequences. Therefore, developers must implement strong data protection measures and ensure transparency regarding the use of emotional data.
Additionally, there is the concern of emotional manipulation, where games might change based on the detection of player emotions to elicit specific responses or encourage certain behaviors. This raises questions about player autonomy and consent, making it crucial for developers to consider the ethical implications of their design choices.
Can emotion detection technology improve player engagement?
Yes, when implemented effectively, emotion detection technology has the potential to significantly enhance player engagement. By adapting game challenges and narratives to the player’s emotional state, games can offer a more personalized and immersive experience. This can lead to increased player satisfaction and retention, as games can dynamically respond to and engage players on a deeper emotional level.
However, the success of such implementations depends on the accuracy of the emotion detection technology and the game’s ability to appropriately respond to the detected emotions. Developers must carefully design these systems to ensure they enrich the gaming experience without overwhelming or alienating players.
Future Predictions for Emotion Detection in Gaming
As we look to the future, several trends suggest how emotion detection technology will evolve in the gaming industry:
- Increased Accuracy and Personalization: Future emotion detection systems will likely achieve greater accuracy and be able to personalize experiences to individual players, enhancing the immersive aspects of games.
- Integration with Virtual Reality: Emotion detection is expected to become a key component in VR gaming, providing more immersive and responsive virtual environments.
- Expansion Beyond Facial Expressions: New technologies may focus on detecting emotions through other means such as physiological responses, broadening the scope of emotion detection.
- Enhanced Player Health Monitoring: Developers could use emotion detection to monitor player well-being, potentially pausing or altering gameplay to prevent distress or negative emotional impacts.
- Regulatory and Ethical Development: As the technology matures, we can anticipate more robust regulatory frameworks to ensure the ethical use of emotion detection in gaming.
More Information
- Discover the stupidity of AI emotion recognition with this little browser game – The Verge: An article discussing the limitations and inaccuracies of AI-based emotion recognition.
- IEEE Xplore Document: A scholarly article on the technical aspects of emotion recognition systems.
- Emotion Recognition for Affect Aware Video Games | SpringerLink: A book chapter that explores the application of emotion recognition in video games.
- ScienceDirect: A research article on multimodal emotion recognition, discussing various methods and their implications.
Disclaimer
This article is generated by AI for educational purposes and does not intend to provide specific advice or recommendations for any individual or specific situation. It is intended to foster exploration and a deeper understanding of the subject matter. Readers are encouraged to conduct further research and consult professionals before implementing any of the technologies or concepts discussed herein.
- Analyzing Patterns in Failed Products - July 25, 2024
- Hybrid Cryptographic Systems - July 24, 2024
- Inadequate Threat Intelligence Integration - July 23, 2024