Overview of Cross-Platform Multiplayer Game Development
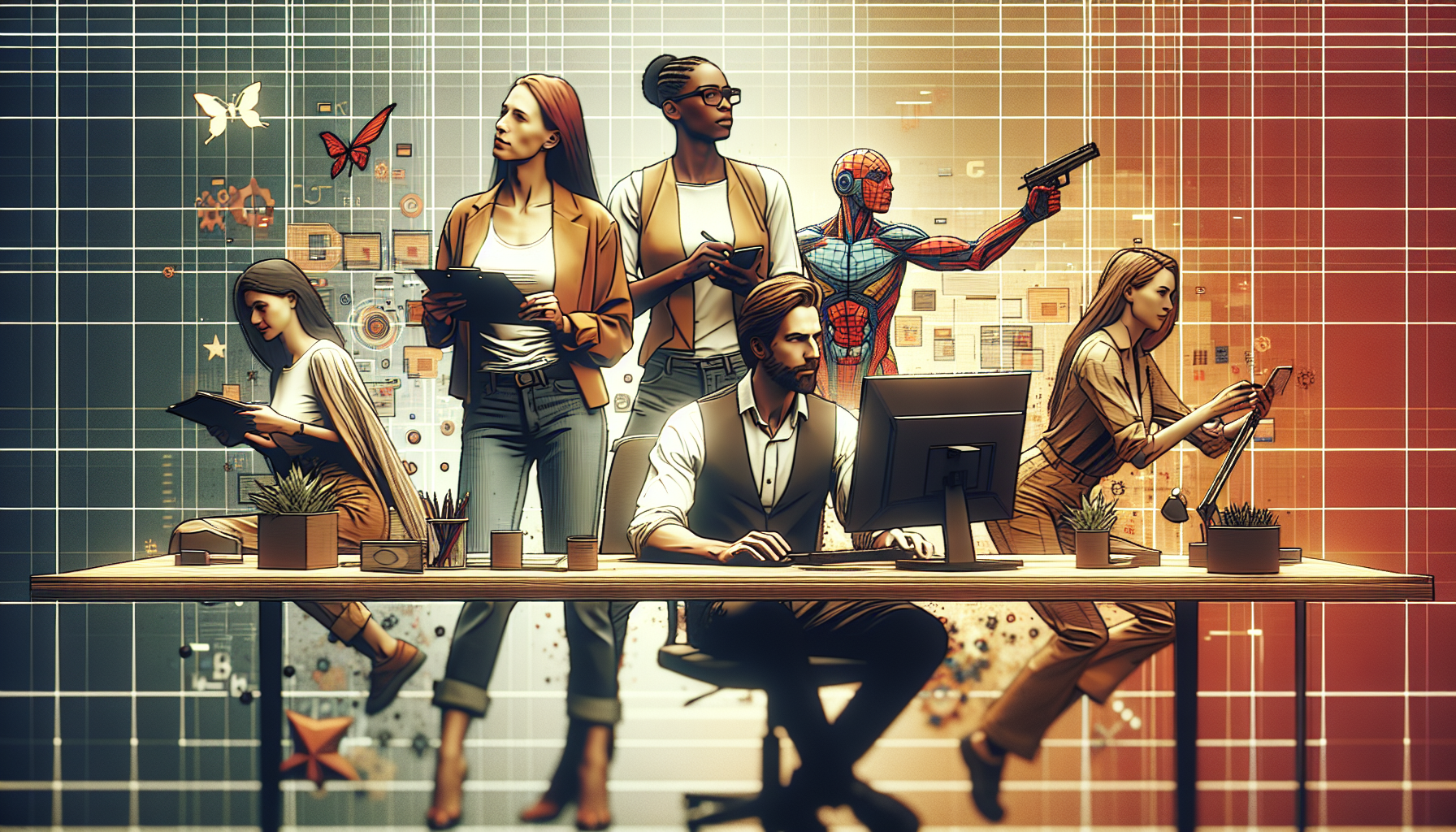
Key Points
- Cross-platform support is increasingly crucial in video games, with major titles like Call of Duty: Warzone 2.0 and Fortnite leading the trend.
- Games like Fallout 4 are receiving updates to enhance cross-platform play capabilities even on newer consoles like the PlayStation 5 and Xbox Series X.
- Microsoft’s Play Anywhere initiative promotes cross-platform play between Xbox and PC, enhancing accessibility and continuity.
- The variety of cross-platform support can vary, with some games offering full cross-platform functionality and others limited to specific combinations of platforms.
- Continuous updates from developers are necessary to maintain and expand cross-platform capabilities.
Technical Foundations
Developing a cross-platform multiplayer game requires a robust technical foundation. This involves selecting a game engine that supports multiple platforms, such as Unity or Unreal Engine. These engines provide tools and libraries that simplify the development process across different hardware specifications.
Networking is another critical component. Developers must implement reliable network code that can handle the demands of synchronous multiplayer games. This includes managing latency and ensuring that the game state is consistent across all platforms.
Lastly, testing is vital. Cross-platform games must be rigorously tested on all supported platforms to ensure a uniform experience. This includes testing user interfaces, graphics performance, and gameplay mechanics.
Challenges and Considerations
One of the main challenges in cross-platform development is dealing with the different capabilities of each platform. For example, a game on a mobile device may not handle graphics as powerfully as a game on a gaming console or PC. Developers must find a balance in game design that offers a good experience on all platforms.
Another consideration is the online infrastructure. Developers need to create or utilize a backend that supports cross-platform play. This often involves complex server management and data synchronization across different operating systems and hardware.
Finally, user account management can be challenging. Players expect to access their profiles and progress across different platforms seamlessly. Implementing a unified account system that is secure and user-friendly is crucial for a successful cross-platform game.
The Challenge of Synchronizing Game State Across Platforms
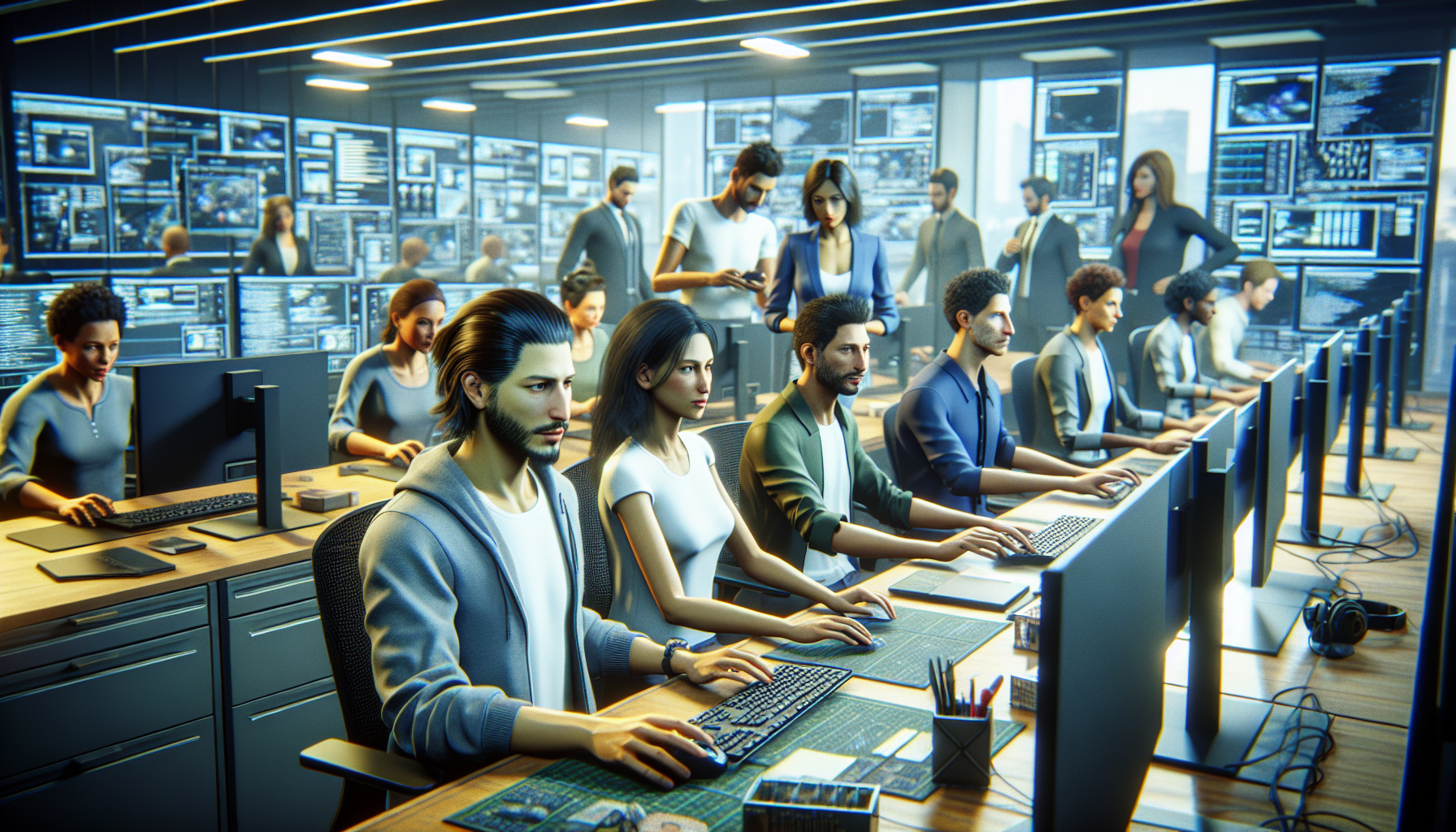
Understanding the Issue
Developers face one of the most significant challenges in cross-platform multiplayer game development: ensuring synchronization of the game state across all platforms. This challenge is crucial because it directly impacts gameplay fairness and user experience.
Discrepancies in game state synchronization can cause problems such as players seeing different game environments or actions registering differently on various platforms. These issues can frustrate players and put them at a competitive disadvantage.
Impact on Gameplay
Poor synchronization can drastically affect gameplay quality. For instance, in a fast-paced shooter game, a slight delay or error in synchronizing player movements can result in unfair deaths or advantages. This not only impacts player satisfaction but can also harm the game’s reputation and player retention.
Moreover, inconsistent game states can complicate gameplay mechanics and interactions within the game, leading to bugs and glitches that can be difficult to diagnose and fix, especially when they only occur under specific platform combinations.
Technical Barriers
Addressing synchronization issues involves overcoming several technical barriers. These include handling latency differences inherent in various networks and optimizing code to run efficiently on diverse hardware. Additionally, developers must implement robust error-checking and correction protocols to handle discrepancies in real-time.
Another technical challenge is the integration of different platform-specific services, such as Xbox Live and PlayStation Network, which may have unique requirements for data handling and user authentication that need to be harmonized within the game’s architecture.
Solutions for Synchronizing Game State
Step 1: Implementing a Robust Networking Layer
The first step in ensuring a consistent game state across platforms is to develop a robust networking layer. This layer should efficiently handle data transmission and include mechanisms for compensating for network latency and packet loss.
Using reliable UDP (User Datagram Protocol) libraries, such as ENet or Lidgren Network, can help in creating a networking layer that is optimized for high-performance multiplayer games.
Step 2: State Management Techniques
The next step involves implementing advanced state management techniques. Techniques such as state interpolation and reconciliation can help in smoothing out discrepancies that arise due to network delays.
State interpolation allows the game to predict and adjust the game state based on the most recent inputs received from different players, while reconciliation involves correcting the game state on a client after receiving the authoritative state from the server.
Step 3: Regular Testing and Optimization
Regular testing across all platforms is essential to identify and fix synchronization issues. This should include stress testing the game under various network conditions to ensure that the networking layer and state management techniques are robust enough.
Optimization may also involve tweaking the game’s engine and codebase to ensure that it performs well on all targeted platforms, thereby minimizing platform-specific discrepancies in gameplay.
Code Example: Synchronizing Game State in Unity
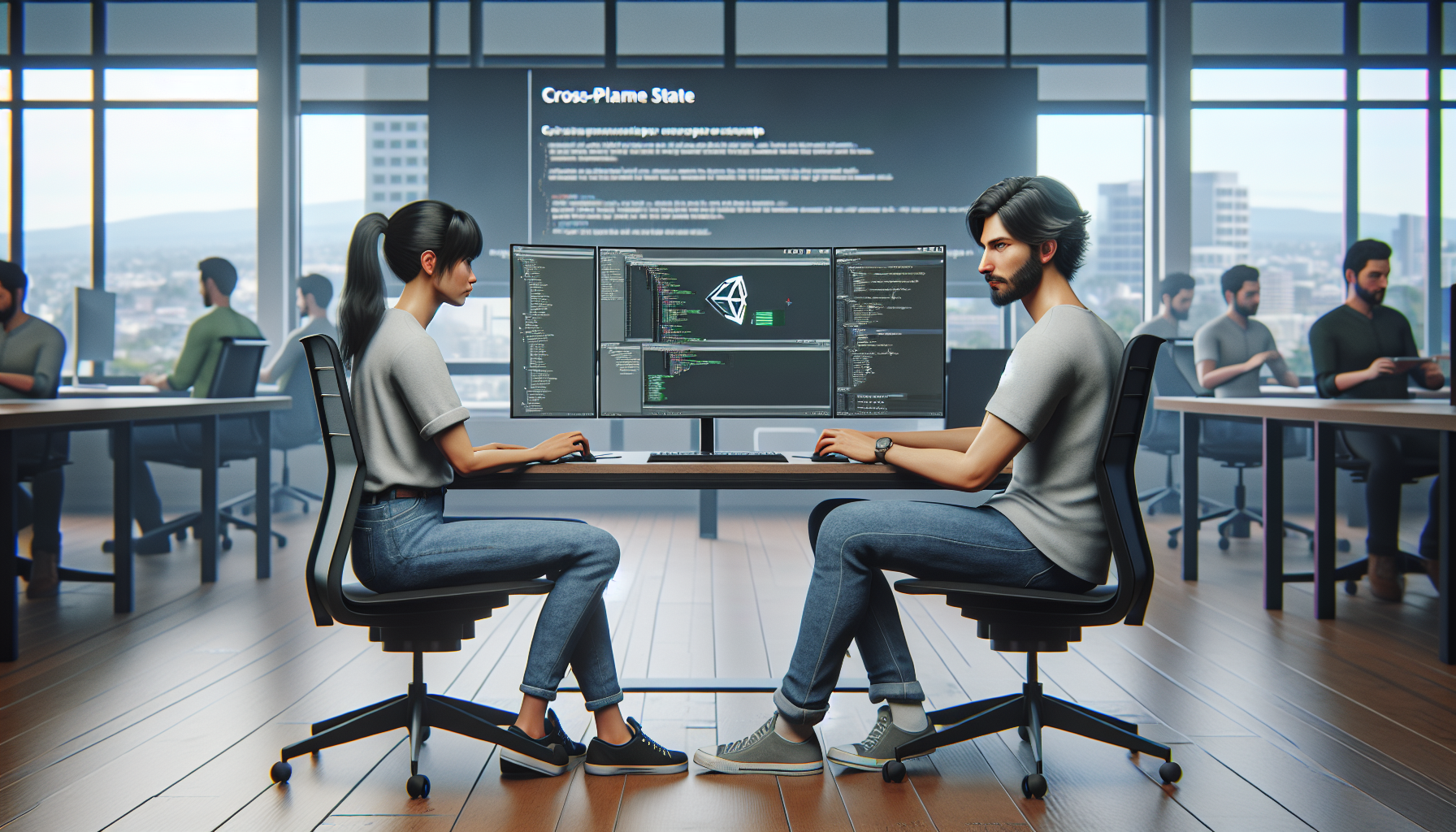
This section provides a practical example of how to synchronize game state across different platforms using Unity, a popular game development engine.
// Unity C# script to synchronize player positions in a multiplayer game public class PlayerSync : MonoBehaviour { private Vector3 lastPosition; private Quaternion lastRotation; private float positionThreshold = 0.1f; private float rotationThreshold = 0.1f; void Update() { if (Vector3.Distance(transform.position, lastPosition) > positionThreshold || Quaternion.Angle(transform.rotation, lastRotation) > rotationThreshold) { lastPosition = transform.position; lastRotation = transform.rotation; NetworkManager.Instance.SendPlayerState(transform.position, transform.rotation); } } } public class NetworkManager { public void SendPlayerState(Vector3 position, Quaternion rotation) { // Code to send the player's state to the server or other players } }
The above code snippet shows a simple implementation in Unity where the player’s position and rotation are monitored. If the position or rotation changes significantly (beyond a set threshold), the new state is sent to other players or the server to update the game state across all clients.
Frequently Asked Questions
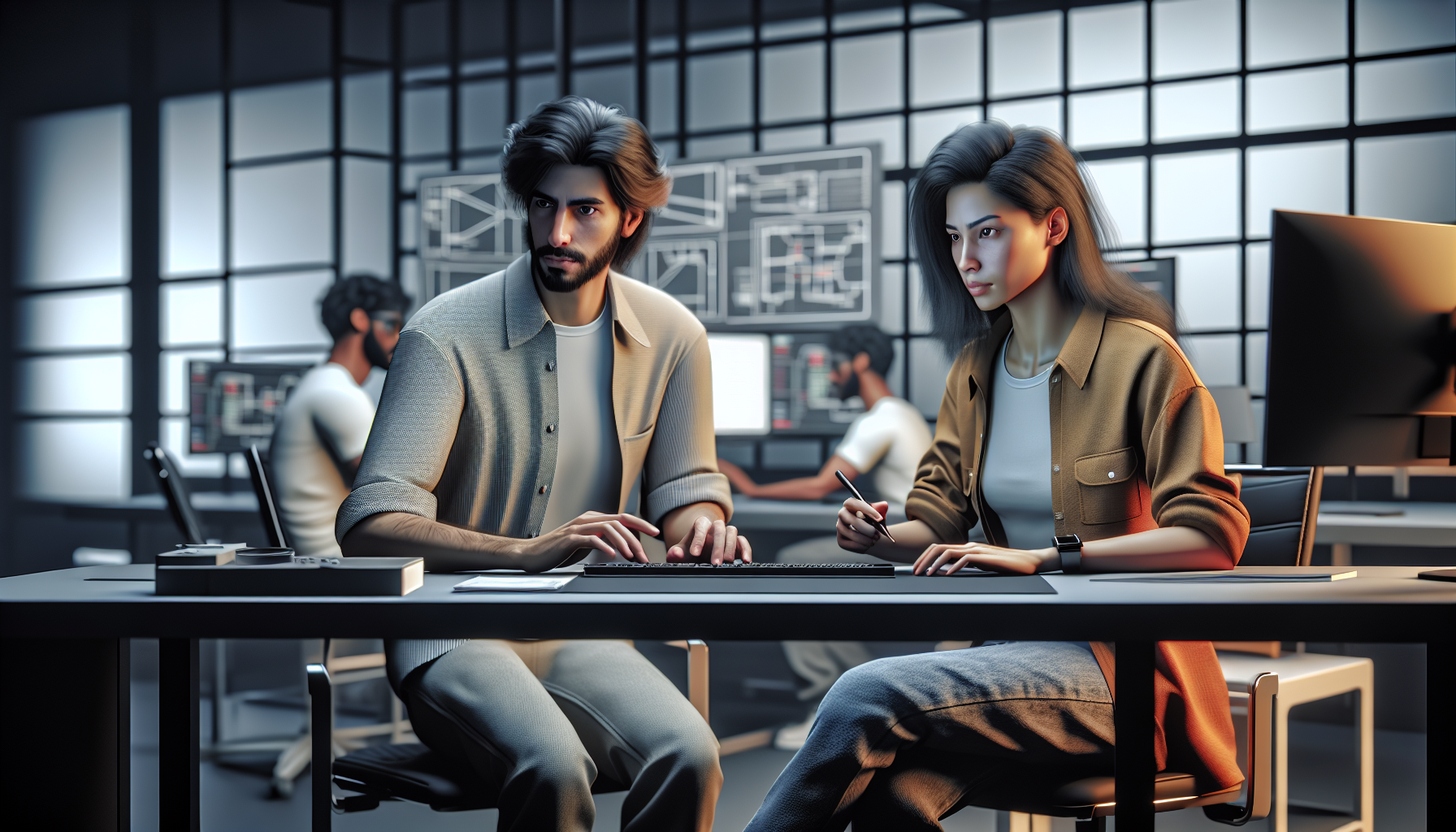
What is cross-platform multiplayer game development?
Cross-platform multiplayer game development involves creating games that players can play together regardless of the gaming platform they are using. This can include combinations of PCs, consoles, and mobile devices.
The goal is to provide a seamless gaming experience where players can interact and compete with each other without being limited by their choice of hardware.
What are the benefits of cross-platform multiplayer games?
One major benefit is the expanded player base. By allowing players from different platforms to interact, developers can significantly increase the potential audience for their game.
Additionally, cross-platform games can lead to longer player retention rates as friends who own different systems can still play together, keeping the game’s community vibrant and active.
What technologies are used in cross-platform multiplayer game development?
Technologies commonly used include networking libraries like Photon, PlayFab, and Unity Networking, which facilitate the development of multiplayer features that work across different platforms.
Game engines such as Unity and Unreal Engine are also pivotal, as they provide the necessary tools to develop and deploy games on multiple platforms.
How do you handle security concerns in cross-platform multiplayer games?
Security is a critical aspect, especially when dealing with player data across different platforms. Implementing robust authentication and encryption protocols is essential to protect user data and prevent cheating.
Regular security audits and updates are also crucial to address any vulnerabilities that may arise and ensure that the game environment remains safe and fair for all players.
Looking Ahead: The Future of Cross-Platform Multiplayer Games
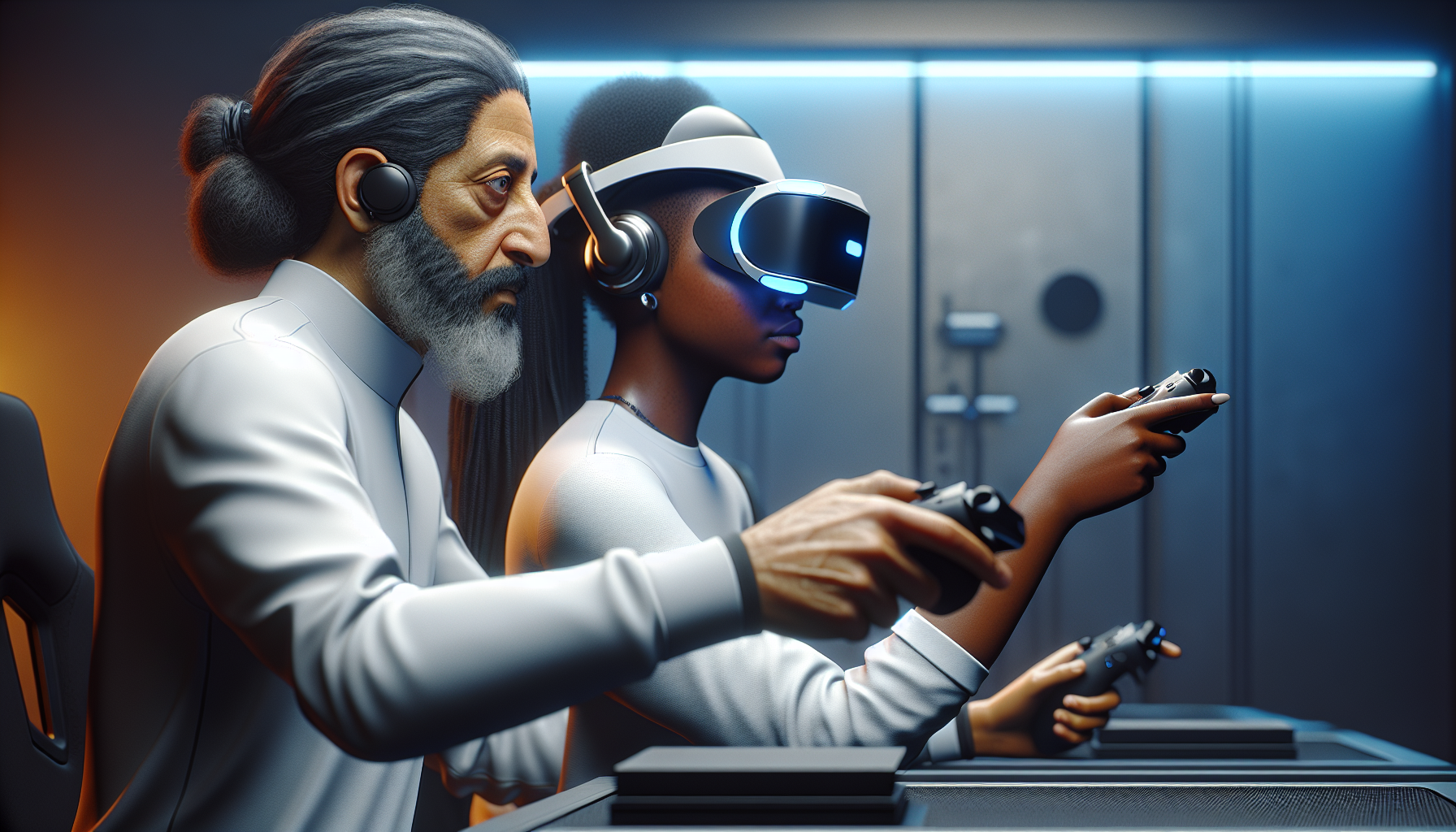
As technology advances, the future of cross-platform multiplayer games looks promising. Here are five predictions based on current trends:
- Increased Adoption of Cloud Gaming: Cloud platforms like Google Stadia and Microsoft xCloud are set to reduce hardware limitations, making cross-platform play even more seamless.
- Advancements in Network Technology: With the rollout of 5G, faster and more reliable internet connections will enhance the multiplayer experience across different devices.
- More Sophisticated Synchronization Techniques: New algorithms and methods for synchronizing game state will emerge, reducing latency issues and improving gameplay fairness.
- Expansion of Cross-Platform Capabilities: More games will support cross-platform features, not just in multiplayer but also in shared progress and purchases across platforms.
- Enhanced Focus on Security: As cross-platform play grows, so will the focus on security, ensuring that multiplayer environments are safe from data breaches and cheating.
More Information
- All cross-platform games (PS5, Xbox Series X, Switch, PC, and more) | Digital Trends
- Any good cross-platform multiplayer games? | Reddit article
- Cross-platform multiplayer? – | Steam Community
- Is the multiplayer cross-platform? Reddit | article
- Cross Platform Multiplayer Console & PC | Steam article
Disclaimer
This article is AI-generated for educational purposes and does not intend to provide specific advice or recommend its implementation. It aims to inspire further research and exploration into the topics covered.
- Analyzing Patterns in Failed Products - July 25, 2024
- Hybrid Cryptographic Systems - July 24, 2024
- Inadequate Threat Intelligence Integration - July 23, 2024